Components
Reusable components created with Bootstrap v3 and integrated with server side via tag library!
Overview
JSmart carries a variety of built-in action based components with server side integration in order to allow creation of web application pretty, easy and fast. All components are integrated with Bootstrap v3 along with all recommendations for developing responsive, mobile first web application projects.
To start using the components in your web application you must use JSP pages and include the following tag lib declaration at the top of your .jsp file.
<%@ taglib prefix="sm" uri="http://jsmartframework.com/v2/jsp/taglib/jsmart" %>
By including the tab lib above, your page will now be able to declare components using the tag
lib sm
as the following example:
<sm:output>Output value</sm:output>
Expression language
To integrate the components with WebBean on server
side you must specify an expression wrapped with @{...}
to retrieve and/or sending
the desired value, as following:
<sm:output value="@{outputBean.anyValue}" />
In the case above the WebBean named as outputBean must provide the getter method getAnyValue to return an object value which will be used on expression analysis.
For components which send values from page such as input, select, checkbox, etc, the WebBean must provide the methods getter and setter for retrieving and send values respectively. The component attributes shall look like as the following example:
<sm:input value="@{inputBean.anyInput}" />
For expression language to provide internationalized text content, it works the same way. So,
for message files mapped via webConfig.xml you must
specify the property name on expression. For instance the texts_en.properties or
texts_pt_BR.properties
message files can be declared via expression language as
following:
<sm:output value="@{texts.my.key}" />
Other uses of expression language include the possibility of expression analysis resulting on if
statement evaluation or even in math calculus. Check this link
http://docs.oracle.com/javaee/1.4/tutorial/doc/JSPIntro7.html#wp77083 to find a variety
of options available for the expression language, but remember that for JSmart components the
expression must be wrapped with @{...}
instead of ${...}
. Check some
examples below:
<sm:output value="@{outputBean.valueOne - outputBean.valueTwo} = @{outputBean.result}"/>
<sm:output value="@{outputBean.valueOne == outputBean.valueTwo ? outputBean.valueThree : outputBean.valueFour}"/>
For action execution on server side via WebBean method calling, the expression must contain the method name on expression such as the following example:
<sm:button action="@{buttontBean.myMethodCall}" />
For more details about WebBean, message property files and webConfig.xml, please have a look at Documentation page.
Output components
JSmart provide a variety of output components for bringing dynamic content from server with useful features and pretty styled elements provided via Bootstrap integration.
Label
Why use it?
Label component can be used to provide dynamic or static content from server side via Expression Language or hardcoded text. The returned value can be internationalized if using resource properties.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled labeling component to your page.
What use inside it?
This component support inner static texts along with other components to provide more functionality such as bind events, Ajax request, icons and tooltip. Have a look in the following components for more details.
Label text
Label text
Label text
Label text
Label from bean
Label text
Label text
Label text with param (one value)
Label text with param (one value)
<sm:label value="Label text" />
<sm:label look="info">Label text</sm:label>
<sm:label value="Label text" target="target-id" />
<sm:label value="Label text" style="font-size: 14px;" look="primary" />
<sm:label value="@{labelBean.label}" style="font-size: 14px;" look="@{labelBean.look}">
<sm:icon name="glyphicon-fire" />
</sm:label>
<sm:label value="Label text" style="font-size: 14px;" look="warning" />
<sm:label value="Label text" style="font-size: 14px;" look="danger">
<sm:tooltip title="Label tooltip" side="top" />
</sm:label>
<sm:label value="Label text with param {0}" style="font-size: 14px;" look="info">
<sm:tooltip title="Label tooltip" side="top" />
<sm:param name="one" value="(one value)"/>
</sm:label>
<sm:label style="font-size: 14px;">
Label text with param %s
<sm:icon name="glyphicon-fire" />
<sm:tooltip title="Label tooltip" side="bottom" />
<sm:param name="one" value="(one value)"/>
</sm:label>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the label component |
style | string | false | - | Specifies the css style to be applied to label component along with Bootstrap label styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to label component along with Bootstrap label styles. It accepts Expression language and/or text values |
target | string | false | - | Specifies target element where this label is semantically assigned for. It accepts Expression language and/or text values |
value | string | false | - | Specifies the value for the label to be show as label text. It accepts Expression language and/or text values |
look | string | false | default | Specifies the Bootstrap look for the label. The possible values are primary,
success, info, warning, danger and default . It accepts Expression language and/or text
values
|
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Output
Why use it?
Output component can be used to provide dynamic or static content from server side via Expression Language or hardcoded text. The returned value can be internationalized if using resource properties.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled output component to your page.
What use inside it?
This component support inner static texts along with other components to provide more functionality such as bind events, Ajax request, icons and tooltip. Have a look in the following components for more details.
Is there another way to use it?
The output component can be also used without tag output
, i.e., you can specify
only the Expression Language such as @{...}
, but in this case only text is
returned.
2 = 2
2 = 2
Some output text
Output value
Output lo ...
Output value
Output long value to be aligned in the center of example
Output long value to be aligned in the right side of example
Output value
Output value parametrized with param (one value) and param (two value)
Output value parametrized with param (one value) and param (two value)
<p>@{outputBean.valueOne - outputBean.valueTwo} = @{outputBean.result}</p>
<sm:output type="p" value="@{outputBean.valueOne - outputBean.valueTwo} = @{outputBean.result}" />
<sm:output id="output-id" type="p">Some output text</sm:output>
<sm:output type="p" value="Output value" transform="uppercase" />
<sm:output type="p" value="Output long value" length="13" ellipsize="true" />
<sm:output type="p" look="info">
<sm:icon name="glyphicon-fire" />
Output value
</sm:output>
<sm:output type="p" look="danger" align="center">
<sm:icon name="glyphicon-fire" />
Output long value to be aligned in the center of example
</sm:output>
<sm:output type="p" look="success" align="right">
<sm:icon name="glyphicon-fire" />
Output long value to be aligned in the right side of example
</sm:output>
<sm:output type="p" look="warning">
Output value
<sm:icon name="glyphicon-fire" />
</sm:output>
<sm:output type="p" look="muted">
Output value parametrized with param {0} and param {1}
<sm:icon name="glyphicon-fire" />
<sm:param name="one" value="(one value)"/>
<sm:param name="two" value="(two value)"/>
</sm:output>
<sm:output type="p" look="info" value="Output value parametrized with param %s and param %s">
<sm:icon name="glyphicon-fire" />
<sm:param name="one" value="(one value)"/>
<sm:param name="two" value="(two value)"/>
</sm:output>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the output component |
style | string | false | - | Specifies the css style to be applied to output along with Bootstrap text styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to output along with Bootstrap text styles. It accepts Expression language and/or text values |
length | integer | false | - | Specifies the maximum length before trimming the output text. It applies only for text provided via value attribute |
ellipsize | boolean | false | - | Specifies if the output text will receive the ... characters at the end
of text after is has been trimmed
|
align | string | false | - | Specifies the output text alignment. Possible values are left, right, center,
justified
|
transform | string | false | - | Specifies transformation to be applied to output text via css. Possible values are:
capitalize, uppercase, lowercase
|
type | string | false | span | Specifies type of the HTML tag element that will wrap the output text. Possible
values are legent, strong, mark, em, small, label, output, del, s, ins, u, p,
h1, h2, h3, h4, h5, h6 and span
|
target | string | false | - | Specifies target element where this output is semantically assigned for. It accepts Expression language and/or text values |
value | string | false | - | Specifies the value for the output text. It accepts Expression language and/or text values |
look | string | false | default | Specifies the Bootstrap look for the output. The possible values are primary,
success, info, warning, danger, muted and default . It accepts Expression language and/or text
values
|
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Format
Why use it?
Format component is meant to be used inside output
or date
components to provide formatted text after component processing, so you can format your
output based on regex or decide the type of your date such as time and or date only.
How to use the regex?
For regex to format Output component with numbers please
check the link
http://docs.oracle.com/javase/7/docs/api/java/text/DecimalFormat.html or for output
date formatting check the link
http://docs.oracle.com/javase/6/docs/api/java/text/SimpleDateFormat.html .
For
regex to format Date component please check the link
http://momentjs.com/docs/#/parsing/string-format/ .
34.39
34.4
12/02/2017
2017.Fevereiro.12 d.C. 09:01 PM
<sm:output type="p" value="@{outputBean.number}">
<sm:format type="number" regex="#.##" />
</sm:output>
<sm:output type="p" value="@{outputBean.number}">
<sm:format type="number" regex="###.#" />
</sm:output>
<sm:output type="p" value="@{outputBean.date}">
<sm:format type="date" regex="dd/MM/YYYY" />
</sm:output>
<sm:output type="p" value="@{outputBean.date}">
<sm:format type="date" regex="yyyy.MMMMM.dd GGG hh:mm aaa" />
</sm:output>
<sm:date value="@{dateBean.date}">
<sm:format type="date" regex="DD / MM / YYYY" />
</sm:date>
<sm:date value="@{dateBean.date}">
<sm:format type="date" regex="MMMM Do YYYY, h:mm:ss a" />
</sm:date>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
type | string | true | - | Specifies the format type for output tag. Possible values are date or
number
|
regex | string | true | - | Specifies regex to be applied on number or date depending on the type
specified. For Output component with number
formatting check the link
http://docs.oracle.com/javase/7/docs/api/java/text/DecimalFormat.html or
for date formatting check the link
http://docs.oracle.com/javase/6/docs/api/java/text/SimpleDateFormat.html to
get the variety of regex options. For Date component with date formatting check the link http://momentjs.com/docs/#/parsing/string-format/ to get the variety of regex options. |
Output list
Why use it?
Output list component can be used to provide dynamic content from server side as undefined list via Expression Language.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled output list component to your page.
What use inside it?
This component do not support inner tags and the values
attribute value must
specify a WebBean instance with attribute of List or Array using Expression language.
- Output item one
- Output item two
- Output item three
- Output item four
- Output item one
- Output item two
- Output item three
- Output item four
- Output item one
- Output item two
- Output item three
- Output item four
- Output item one
- Output item two
- Output item three
- Output item four
- Output item one
- Output item two
- Output item three
- Output item four
- Output item one
- Output item two
- Output item three
- Output item four
<sm:outputlist values="@{outputBean.list}" />
<sm:outputlist values="@{outputBean.list}" look="info" />
<sm:outputlist values="@{outputBean.list}" look="success" />
<sm:outputlist values="@{outputBean.list}" look="danger" />
<sm:outputlist values="@{outputBean.list}" look="warning" inline="true" />
<sm:outputlist values="@{outputBean.list}" look="muted" inline="true" />
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the output list component |
style | string | false | - | Specifies the css style to be applied to output list along with Bootstrap text and list styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to output list along with Bootstrap text and list styles. It accepts Expression language and/or text values |
values | string | true | - | Specifies the values for the output list. It only accepts Expression language and the variable containing the values must an instance of Array or List Collection |
inline | boolean | false | false | Specifies if the list is presented inline or in column mode |
look | string | false | default | Specifies the Bootstrap look for the output list. The possible values are primary,
success, info, warning, danger, muted and default . It accepts Expression language and/or text
values
|
Text
Why use it?
Text component can be used to provide internationalized dynamic content from server side with dynamic parameters specified on resource properties via Expression Language.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled text to your page.
How to use it?
This components must refer the res
attribute value to the resource property
file name as explained in Documentation and the
key
attribute value must refer to a key inside this resource property file.
For parametrized content, the text on the resource property file must contain the
expression {<ordinal>}
or %s
.
The entire text can only
contain one or another character specified above.
Is there another way to use it?
The text component can be also used without tag text
, i.e., you can specify
only the Expression Language such as @{...}
, but in this case only text is
returned and the parameters are not applied.
Simple text via Expression Language only
I got the feeling deeping inside.
Please, believe me I hate to miss the train, ow
yeah.
But if you believe me, I wont be late again ow yeah.
Parameter (Parameter one) I got the feeling deeping inside.
Please, believe me I hate to
miss the train, ow yeah.
But if you believe me, I wont be late again ow yeah. Parameter
(Parameter two).
@{texts.jsmart.components.text.simple}
<sm:text res="texts" key="jsmart.components.text.example" />
<sm:text res="texts" key="jsmart.components.text.example.with.parameters">
<sm:param name="one" value="(Parameter one)"/>
<sm:param name="two" value="(Parameter two)"/>
</sm:text>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
res | string | true | - | Specifies the resource property file name to retrieve the texts. The resource name must be mapped on webConfig.xml according to Documentation |
key | string | true | - | Specifies the key to retrieve the text inside resource property file. |
Badge
Why use it?
Badge component can be used to provide dynamic or static content from server side via Expression Language or hardcoded text. The returned value can be internationalized if using resource properties.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled badge component to your page.
What use inside it?
This component support inner static texts along with other components to provide more functionality such as bind events, Ajax request and tooltip. Have a look in the following components for more details.
Badge label
Badge bean label
Badge bean label
<sm:badge label="Badge label" />
<sm:badge label="@{badgeBean.label}" />
<sm:badge label="@{badgeBean.label}" style="font-size: 20px; background: #ce4844;" />
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the badge component |
style | string | false | - | Specifies the css style to be applied to badge component along with Bootstrap badge styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to badge component along with Bootstrap badge styles. It accepts Expression language and/or text values |
label | string | false | - | Specifies the label for the badge text. It accepts Expression language and/or text values |
Header
Why use it?
Header component can be used to provide dynamic or static content from server side via Expression Language or hardcoded text. The returned value can be internationalized if using resource properties.
Where to use it?
It can be placed anywhere in your JSP page and even inside the following components to provide pretty styled header to wrapping component.
What use inside it?
This component support inner static texts along with other components to provide more functionality such as bind events, Ajax request, and icons. Have a look in the following components for more details.
Header title
Header title
Header title
Header title
Panel header
Panel content here
Alert header
Alert from WebBean
<sm:header title="Header title" type="h5" />
<sm:header title="@{headerBean.title}" />
<sm:header title="Header title" type="h4">
<sm:icon name="glyphicon-fire"/>
</sm:header>
<sm:header title="Header title" type="h4">
<sm:icon name="glyphicon-fire" side="right"/>
</sm:header>
<sm:panel look="info">
<sm:header title="Panel header">
<sm:icon name="glyphicon-thumbs-up"/>
</sm:header>
<p>
Panel content here
</p>
</sm:panel>
<sm:alert id="alert-5">
<sm:header title="Alert header">
<sm:icon name="glyphicon-fire"/>
</sm:header>
</sm:alert>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the header component |
style | string | false | - | Specifies the css style to be applied to header component along with Bootstrap header styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to header component along with Bootstrap header styles. It accepts Expression language and/or text values |
title | string | false | - | Specifies the title for the header text. It accepts Expression language and/or text values |
type | string | false | h3 | Specifies the type for the header component. Possible values are h1, h2, h3,
h4, h5 or h6
|
Footer
Why use it?
Footer component can be used to provide dynamic or static content from server side via Expression Language or hardcoded text. The returned value can be internationalized if using resource properties.
Where to use it?
It can be placed anywhere in your JSP page and even inside the following components to provide pretty styled footer to wrapping component.
What use inside it?
This component support inner static texts along with other component tags, in special the following components to provide more functionality such as bind events and Ajax request. Have a look in the following components for more details.
Panel content here
<sm:footer title="Footer title" />
<sm:footer title="@{footerBean.title}" />
<sm:footer>
<sm:output value="Any footer content here " />
<sm:icon name="glyphicon-fire"/>
</sm:footer>
<sm:panel look="primary">
<p>
Panel content here
</p>
<sm:footer title="Panel footer ">
<sm:icon name="glyphicon-thumbs-up"/>
</sm:footer>
</sm:panel>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the footer component |
style | string | false | - | Specifies the css style to be applied to footer component along with Bootstrap footer styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to footer component along with Bootstrap footer styles. It accepts Expression language and/or text values |
title | string | false | - | Specifies the title for the footer text. It accepts Expression language and/or text values |
Repeat
Why use it?
Repeat component allows you to create repeated components based on a list of objects from server such as dropactions inside dropmenu, panels inside accordion or any other standalone components such as text, button, link, etc.
Where to use it?
It can be placed anywhere in your JSP page and even inside the following components:
What use inside it?
This component support any component tags to be created on an iterable way. It also supports the Empty component to present empty message or complex HTML when repeat values return empty list of objects to iterate over.
Repeat item one
Repeat item two
Repeat item three
Repeat item one Repeat item two Repeat item three
Repeat item one
Repeat item two
Repeat item three
<sm:repeat var="obj" values="@{repeatBean.values}">
<sm:output type="p" value="@{obj}" />
</sm:repeat>
<sm:repeat var="obj" values="@{repeatBean.values}">
<sm:button label="@{obj}" style="margin-right: 15px;" />
</sm:repeat>
<sm:repeat var="obj" values="@{repeatBean.values}">
<sm:link label="@{obj}" style="margin-right: 15px;">
<sm:icon name="glyphicon-globe" />
</sm:link>
</sm:repeat>
<sm:button label="Dropdown label">
<sm:icon name="glyphicon-fire" />
<sm:dropmenu>
<sm:repeat var="obj" values="@{repeatBean.values}">
<sm:dropaction>
<sm:checkbox label="@{obj}" />
</sm:dropaction>
</sm:repeat>
</sm:dropmenu>
</sm:button>
<sm:repeat var="obj" values="@{repeatBean.values}">
<sm:input placeholder="@{obj}" style="margin-bottom: 15px;" leftAddOn="input_addon">
<sm:button id="input_addon" label="@{obj}" />
</sm:input>
</sm:repeat>
<sm:repeat var="obj" values="@{repeatBean.values}">
<sm:panel>
<sm:header title="@{obj}">
<sm:icon name="glyphicon-th-list" />
</sm:header>
<sm:panelbody align="center">
<sm:output value="@{obj}" />
</sm:panelbody>
</sm:panel>
</sm:repeat>
<sm:accordion>
<sm:repeat var="obj" values="@{repeatBean.values}">
<sm:panel>
<sm:header title="@{obj}">
<sm:icon name="glyphicon-th-list" />
</sm:header>
<sm:panelbody align="center">
<sm:output value="@{obj}" />
</sm:panelbody>
</sm:panel>
</sm:repeat>
</sm:accordion>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
var | string | true | - | Specifies the variable name to be used when iterating over repeat inner components. This value must also be used to access each item on provided list to capture the value for informed attribute names on inner tags. |
values | string | true | - | Specifies the attribute exposed via getter method of type List Collection to provided the list of objects to be used while iterating on repeat component to render inner tags. It only accept Expression language values. |
Input components
JSmart provide a variety of input components for sending and retrieving dynamic content to and from server with useful features and pretty styled elements provided via Bootstrap integration.
Input
Why use it?
Input component can be used to easily exchange data with server side via Expression Language to integrate client side with WebBean on server side.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled input component to your page.
What use inside it?
This component support inner components to provide more functionality such as bind events, Ajax request, buttons and tooltip. Have a look in the following components for more details.
How to use it?
To integrate the input
component with WebBean you must specify the Expression language on attribute
value
pointing to WebBean attribute name which must be exposed via getter and
setter methods.
For some type of input the getter can be ignored such as
password
type.
How to place button on right and/or left side?
To place inner button
components aside of the input there are the attributes
leftAddOn
and rightAddOn
, which generally hold text values, but if
they specify the button id the input will replace the text by the button component.
<sm:input>
<sm:popover title="Popover title" content="Popover content" side="top" event="hover" />
</sm:input>
<sm:input label="Input small" size="small" value="@{inputBean.inputValue}" />
<sm:input label="Input label" value="@{inputBean.inputValue}" />
<sm:input label="Password input" type="password" value="@{inputBean.inputValue}" />
<sm:input label="Number input" type="number" value="@{inputBean.inputValue}" />
<sm:input label="Input mask" value="@{inputBean.inputValue}" mask="999-99-999-9999-9" />
<sm:input label="Input placeholder" value="@{inputBean.inputValue}" placeholder="Input placeholder" />
<sm:input label="Input left addon" leftAddOn="Left addon" value="@{inputBean.inputValue}" placeholder="Input placeholder" />
<sm:input leftAddOn="Left addon" value="@{inputBean.inputValue}" placeholder="Input placeholder" rightAddOn="Right addon" />
<sm:input leftAddOn="btn-left-addon" value="@{inputBean.inputValue}" placeholder="Input placeholder">
<sm:button id="btn-left-addon" label="Addon button" look="primary" />
</sm:input>
<sm:input rightAddOn="btn-right-addon" value="@{inputBean.inputValue}" placeholder="Input placeholder">
<sm:button id="btn-right-addon" label="Addon button" look="danger">
<sm:dropmenu segmented="true">
<sm:dropaction label="Button action" />
</sm:dropmenu>
</sm:button>
</sm:input>
<sm:input leftAddOn="btn-left-addon-1" rightAddOn="btn-right-addon-1" value="@{inputBean.inputValue}" placeholder="Input placeholder">
<sm:button id="btn-left-addon-1" label="Left button" look="warning">
<sm:icon name="glyphicon-lamp" />
</sm:button>
<sm:button id="btn-right-addon-1" label="Right button" look="info">
<sm:icon name="glyphicon-apple" />
</sm:button>
</sm:input>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the input component |
style | string | false | - | Specifies the css style to be applied to input component along with Bootstrap input styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to input component along with Bootstrap input styles. It accepts Expression language and/or text values |
type | string | false | - | Specifies the HTML type for the input. Possible values are text, password,
hidden, number, search, range, email, url, date, month, week, time, datetime,
datetime-local, color, tel . The type interferes on component rendering and
its is Browser dependent
|
rest | string | false | - | Specifies the JSON key name or XML tag name for REST requests using Rest component. Check Rest component for more details |
length | integer | false | - | Specifies maximum length allowed for text content on input component |
size | string | false | - | Specifies the Bootstrap size for the input. Possible values are small,
large
|
value | string | false | - | Specifies the value for the input component. It accepts Expression language and/or text values. |
readOnly | boolean | false | - | Specifies if the input component is read only field |
disabled | boolean | false | - | Specifies if the input component is disabled |
placeholder | string | false | - | Specifies the placeholder to be presented inside the input component to easy the field understanding. It accepts Expression language and/or text values |
label | string | false | - | Specifies label for the input component. It accepts Expression language and/or text values |
leftAddOn | string | false | - | It includes an addon button component or static text on left side of the input
component. It accepts Expression
language and/or text values. To include a Button
component as leftAddOn the attribute must contain the same id as the
button tag specified as inner tag of input component
|
rightAddOn | string | false | - | It includes an addon button component or static text on right side of the input
component. It accepts Expression
language and/or text values. To include a Button
component as rightAddOn the attribute must contain the same id as the
button specified as inner tag of input component
|
pattern | string | false | - | Specifies the HTML pattern for the input. The type interferes on component rendering and its is Browser dependent |
autoFocus | boolean | false | - | Specifies if the input component must be focused when page is rendered |
minValue | integer | false | - | Specifies the minimum value for input case its type is range |
maxValue | integer | false | - | Specifies the maximum value for input case its type is range |
stepValue | integer | false | - | Specifies the step value for input case its type is range |
tabIndex | integer | false | - | Specifies the tab index for the input component be focused when page is traversed by tab key |
mask | string | false | - |
Specifies mask to be applied on input text content. Check the possible values on
|
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Select
Why use it?
Select component can be used to present a range of options for selection ready to integrate with server side via Expression Language and WebBean.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled select component to your page.
What use inside it?
This component support inner components to provide more functionality such as options, bind events, Ajax request, buttons and tooltip. Have a look in the following components for more details.
How to use it?
To integrate the select
component with WebBean you must specify the Expression language on attribute selectValues
pointing to WebBean attribute name which must be exposed via getter and setter methods.
For mulitple selection the attribute on WebBean must be the type of List Collection and for
single selection the type of Object.
To fill in the selection options you must include
one or both of the following inner tags
How to place button on right and/or left side?
To place inner button
components aside of the select there are the attributes
leftAddOn
and rightAddOn
, which generally hold text values, but if
they specify the button id the select will replace the text by the button component.
<sm:select>
<sm:option label="Label one" value="value one" />
<sm:option label="Label two" value="value two" />
<sm:option label="Label three" value="value three" />
</sm:select>
<sm:select label="Select small" size="small">
<sm:option label="Label one" value="value one" />
<sm:option label="Label two" value="value two" />
<sm:option label="Label three" value="value three" />
</sm:select>
<sm:select selectValues="@{selectBean.selectValues}" label="Select with option">
<sm:option label="Label one" value="value one" />
<sm:option label="Label two" value="value two" />
<sm:option label="Label three" value="value three" />
</sm:select>
<sm:select selectValues="@{selectBean.selectValues}" label="Select with options">
<sm:options values="@{selectBean.optionValues}" />
</sm:select>
<sm:select selectValues="@{selectBean.selectValues}" label="Select multiple" multiple="true">
<sm:options values="@{selectBean.optionValues}" />
</sm:select>
<sm:select selectValues="@{selectBean.selectValues}" label="Select left addon" leftAddOn="Left addon">
<sm:options values="@{selectBean.optionValues}" />
</sm:select>
<sm:select selectValues="@{selectBean.selectValues}" label="Select right addon" rightAddOn="btn-right-select">
<sm:options values="@{selectBean.optionValues}" />
<sm:button id="btn-right-select" label="Addon button" look="danger">
<sm:dropmenu segmented="true">
<sm:dropaction label="Button action" />
</sm:dropmenu>
</sm:button>
</sm:select>
<sm:select selectValues="@{selectBean.selectValues}" label="Select button addons" leftAddOn="btn-left-select-1"
rightAddOn="btn-right-select-1">
<sm:options values="@{selectBean.optionValues}" />
<sm:button id="btn-left-select-1" label="Left button" look="primary">
<sm:icon name="glyphicon-lamp" />
</sm:button>
<sm:button id="btn-right-select-1" label="Right button" look="danger">
<sm:icon name="glyphicon-apple" />
</sm:button>
</sm:select>
<sm:select selectValues="@{selectBean.selectValues}" label="Select multiple with addons" leftAddOn="Left addon"
rightAddOn="Right addon" multiple="true">
<sm:options values="@{selectBean.optionValues}" />
</sm:select>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the select component |
style | string | false | - | Specifies the css style to be applied to select component along with Bootstrap select styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to select component along with Bootstrap select styles. It accepts Expression language and/or text values |
rest | string | false | - | Specifies the JSON key name or XML tag name for REST requests using Rest component. Check Rest component for more details |
size | string | false | - | Specifies the Bootstrap size for the select. Possible values are small,
large
|
multiple | boolean | false | false | Specifies if the select component accepts multiple selection. In case
true the attribute mapped on WebBean
must be the type of List Collection
|
ajax | boolean | false | - | Specifies if the action to set value on mapped selectValues attribute on WebBean should use Ajax request on select event |
selectValues | string | false | - | Specifies the selected values for the select component as a List Collection for multiple selection or Object for single selection. It accepts Expression language and/or text values. |
disabled | boolean | false | - | Specifies if the select component is disabled |
label | string | false | - | Specifies label for the select component. It accepts Expression language and/or text values |
leftAddOn | string | false | - | It includes an addon button component or static text on left side of the select
component. It accepts Expression
language and/or text values. To include a Button
component as leftAddOn the attribute must contain the same id as the
button tag specified as inner tag of select component
|
rightAddOn | string | false | - | It includes an addon button component or static text on right side of the select
component. It accepts Expression
language and/or text values. To include a Button
component as rightAddOn the attribute must contain the same id as the
button specified as inner tag of select component
|
tabIndex | integer | false | - | Specifies the tab index for the select component be focused when page is traversed by tab key |
update | string | false | - | Specifies the id of elements to be updated after Ajax request completes. The ids must be specified as comma separated values so the HTML elements can be update with Ajax request response content. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the Ajax request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Option
Why use it?
Option component is meant to be used as inner tag of select component to provide a single option with label and value for select component.
Where to use it?
The option component only takes effect inside the Select component. Check some examples on select component section.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the option component |
style | string | false | - | Specifies the css style to be applied to option component along with Bootstrap option styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to option component along with Bootstrap option styles. It accepts Expression language and/or text values |
value | string | true | - | Specifies the option value for the select component. It accepts Expression language and/or text values |
label | string | true | - | Specifies the option label for the select component. It accepts Expression language and/or text values |
disabled | boolean | false | - | Specifies if the select option is disabled |
Options
Why use it?
Options component is meant to be used as inner tag of select component to provide a Map of options with label and value for select component.
Where to use it?
The options component only takes effect inside the Select component. Check some examples on select component section.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
values | string | true | - | Specifies the option values for the select component as a Map Collection containing key/value pairs. Map key is for option value and Map value is for option label. It only accepts Expression language to map WebBean attribute to provide the Map collection |
Checkbox
Why use it?
Checkbox component can be used to easily exchange boolean value with server side via Expression Language to integrate client side with WebBean on server side.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled checkbox component to your page.
What use inside it?
This component support inner components to provide more functionality such as bind events, Ajax request and tooltip. Have a look in the following components for more details.
How to use it?
To integrate the checkbox
component with WebBean you must specify the Expression language on attribute
value
pointing to WebBean Boolean attribute name which must be exposed via
getter and setter methods.
<sm:checkbox label="Simple checkbox" />
<sm:checkbox label="Integrated checkbox" value="@{checkboxBean.checked}" />
<sm:checkbox id="checkbox" ajax="true" label="Ajax checkbox" value="@{checkboxBean.checked}" />
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the checkbox component |
style | string | false | - | Specifies the css style to be applied to checkbox component along with Bootstrap checkbox styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to checkbox component along with Bootstrap checkbox styles. It accepts Expression language and/or text values |
rest | string | false | - | Specifies the JSON key name or XML tag name for REST requests using Rest component. Check Rest component for more details |
value | string | false | - | Specifies the value for the checkbox component as Boolean object. It accepts Expression language and/or text values. |
tabIndex | integer | false | - | Specifies the tab index for the checkbox component be focused when page is traversed by tab key |
disabled | boolean | false | - | Specifies if the checkbox component is disabled |
label | string | false | - | Specifies label for the checkbox component. It accepts Expression language and/or text values |
ajax | boolean | false | - | Specifies if the action to set value on mapped value attribute on WebBean should use Ajax request on click event |
update | string | false | - | Specifies the id of elements to be updated after Ajax request completes. The ids must be specified as comma separated values so the HTML elements can be update with Ajax request response content. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the Ajax request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Radio group
Why use it?
Radio group component can be used to present a range of check items for selection ready to integrate with server side via Expression Language and WebBean.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled radio group component to your page.
What use inside it?
This component support inner components to provide more functionality such as check, bind events, Ajax request and tooltip. Have a look in the following components for more details.
How to use it?
To integrate the radiogroup
component with WebBean you must specify the Expression language on attribute selectValue
pointing to WebBean attribute name with type of Object which must be exposed via getter and
setter methods.
To fill in the radiogroup items you must include one or both of the
following inner tags
<sm:radiogroup label="Column radio group">
<sm:check label="Label one" value="value_one" />
<sm:check label="Label two" value="value_two" />
<sm:check label="Label three" value="value_three" />
</sm:radiogroup>
<sm:radiogroup label="Inline radio group" inline="true">
<sm:check label="Label one" value="value_one" />
<sm:check label="Label two" value="value_two" />
<sm:check label="Label three" value="value_three" />
</sm:radiogroup>
<sm:radiogroup id="radio" ajax="true" label="Select radio group" inline="true"
selectValue="@{radioBean.selectedValue}">
<sm:checklist values="@{radioBean.radioValues}" />
<sm:check label="Label Five" value="value_five" />
</sm:radiogroup>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the radio group component |
style | string | false | - | Specifies the css style to be applied to radio group component along with Bootstrap radio styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to radio group component along with Bootstrap radio styles. It accepts Expression language and/or text values |
rest | string | false | - | Specifies the JSON key name or XML tag name for REST requests using Rest component. Check Rest component for more details |
inline | boolean | false | false | Specifies if radio group items will be presented in column or inline. Possible
values are true, false
|
disabled | boolean | false | - | Specifies if the radiogroup component is disabled |
align | string | false | - | Specifies the radio group check items alignment. Possible values are left,
right, center
|
selectValue | string | false | - | Specifies the selected value for the radio group component as a Object. It accepts Expression language and/or text values. |
disabled | boolean | false | - | Specifies if the radio group component is disabled |
label | string | false | - | Specifies label for the radio group component. It accepts Expression language and/or text values |
ajax | boolean | false | - | Specifies if the action to set value on mapped selectValue attribute on WebBean should use Ajax request on click event |
update | string | false | - | Specifies the id of elements to be updated after Ajax request completes. The ids must be specified as comma separated values so the HTML elements can be update with Ajax request response content. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the Ajax request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Check group
Why use it?
Check group component can be used to present a range of check items for multiple selection ready to integrate with server side via Expression Language and WebBean.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled check group component to your page.
What use inside it?
This component support inner components to provide more functionality such as check, bind events, Ajax request and tooltip. Have a look in the following components for more details.
How to use it?
To integrate the checkgroup
component with WebBean you must specify the Expression language on attribute selectValues
pointing to WebBean attribute name with type of Object which must be exposed via getter and
setter methods.
To fill in the checkgroup items you must include one or both of the
following inner tags
<sm:checkgroup label="Column check group">
<sm:check label="Label one" value="value_one" />
<sm:check label="Label two" value="value_two" />
<sm:check label="Label three" value="value_three" />
</sm:checkgroup>
<sm:checkgroup label="Inline check group" inline="true">
<sm:check label="Label one" value="value_one" />
<sm:check label="Label two" value="value_two" />
<sm:check label="Label three" value="value_three" />
</sm:checkgroup>
<sm:checkgroup id="checkgroup" ajax="true" label="Select check group" inline="true"
selectValues="@{checkGroupBean.selectedValues}">
<sm:checklist values="@{checkGroupBean.checkValues}" />
<sm:check label="Label Five" value="value_five" />
</sm:checkgroup>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the check group component |
style | string | false | - | Specifies the css style to be applied to check group component along with Bootstrap checkbox styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to check group component along with Bootstrap checkbox styles. It accepts Expression language and/or text values |
rest | string | false | - | Specifies the JSON key name or XML tag name for REST requests using Rest component. Check Rest component for more details |
inline | boolean | false | false | Specifies if check group items will be presented in column or inline. Possible
values are true, false
|
disabled | boolean | false | - | Specifies if the checkgroup component is disabled |
align | string | false | - | Specifies the check group check items alignment. Possible values are left,
right, center
|
selectValues | string | false | - | Specifies the selected values for the checkgroup component as a List Collection for multiple selection. It accepts Expression language and/or text values. |
disabled | boolean | false | - | Specifies if the check group component is disabled |
label | string | false | - | Specifies label for the check group component. It accepts Expression language and/or text values |
ajax | boolean | false | - | Specifies if the action to set value on mapped selectValues attribute on WebBean should use Ajax request on click event |
update | string | false | - | Specifies the id of elements to be updated after Ajax request completes. The ids must be specified as comma separated values so the HTML elements can be update with Ajax request response content. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the Ajax request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Check
Why use it?
Check component is meant to be used as inner tag of radiogroup
or checkgroup
components to provide a single check with label and value for wrapper component.
Where to use it?
The check component only takes effect inside the Radio group or Check group components. Check some examples on those component component sections.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
value | string | true | - | Specifies the check value for the radiogroup or
checkgroup component. It accepts Expression language and/or text
values
|
label | string | true | - | Specifies the check label for the radiogroup or
checkgroup component. It accepts Expression language and/or text
values
|
disabled | boolean | false | - | Specifies if the check item is disabled |
Checklist
Why use it?
Checklist component is meant to be used as inner tag of radiogroup
or checkgroup
components to provide a Map of items with label and value for those components.
Where to use it?
The checklist component only takes effect inside the Radio group or Check group component. Check some examples on those component sections.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
values | string | true | - | Specifies the check item values for the radiogroup or
checkgroup components as a Map Collection containing key/value pairs.
Map key is for check item value and Map value is for check item label. It only
accepts Expression language to map WebBean attribute to provide the
Map collection
|
Date
Why use it?
Date component can be used to easily provide a calendar for date picking and exchange date with server via Expression Language to integrate client side with WebBean on server side.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled date component to your page.
What use inside it?
This component support inner components to provide more functionality such as format, bind events, Ajax request, buttons and tooltip. Have a look in the following components for more details.
How to use it?
To integrate the date
component with WebBean
you must specify the Expression language on
attribute value
pointing to WebBean attribute name which must be exposed via
getter and setter methods.
The date
component integrates with String, Date
util or Date from Joda time, so the WebBean
can expose one of the three options via Expression Language.
How to place button on right and/or left side?
To place inner button
components aside of the date there are the attributes
leftAddOn
and rightAddOn
, which generally hold text values, but if
they specify the button id the date component will replace the text by the button component.
<sm:date label="Simple date" />
<sm:date label="Integrated date" placeholder="Enter the date placeholder" value="@{dateBean.date}" />
<sm:date label="Date with locale" value="@{dateBean.date}" locale="fr" />
<sm:date label="Date showing weeks" value="@{dateBean.date}" showWeeks="true" />
<sm:date label="Date mode days" value="@{dateBean.date}" dateMode="days" />
<sm:date label="Date mode months" value="@{dateBean.date}" dateMode="months" />
<sm:date label="Date mode time only" value="@{dateBean.date}" dateMode="timeonly" />
<sm:date label="Date with left addon" value="@{dateBean.date}" leftAddOn="Left addon" />
<sm:date label="Date with right addon" value="@{dateBean.date}" rightAddOn="Right addon" />
<sm:date label="Date with right addon button" value="@{dateBean.date}" rightAddOn="date-btn-id">
<sm:button id="date-btn-id" label="Button" look="primary" />
</sm:date>
<sm:date label="Date with format" value="@{dateBean.date}">
<sm:format type="date" regex="MMMM Do YYYY, h:mm:ss a" />
</sm:date>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the date component |
style | string | false | - | Specifies the css style to be applied to date component along with Bootstrap date styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to date component along with Bootstrap date styles. It accepts Expression language and/or text values |
rest | string | false | - | Specifies the JSON key name or XML tag name for REST requests using Rest component. Check Rest component for more details |
size | string | false | - | Specifies the Bootstrap size for the date input. Possible values are small,
large
|
value | string | false | - | Specifies the value for the date component. It accepts Expression language and/or text
values. Date component can be integrated with String, Date util or Date Joda time on WebBean attribute declared via Expression Language |
readOnly | boolean | false | - | Specifies if the date input component is read only field |
disabled | boolean | false | - | Specifies if the date input component is disabled |
placeholder | string | false | - | Specifies the placeholder to be presented inside the date input component to easy the field understanding. It accepts Expression language and/or text values |
label | string | false | - | Specifies label for the date component. It accepts Expression language and/or text values |
leftAddOn | string | false | - | It includes an addon button component or static text on left side of the date input
component. It accepts Expression
language and/or text values. To include a Button
component as leftAddOn the attribute must contain the same id as the
button tag specified as inner tag of input component
|
rightAddOn | string | false | - | It includes an addon button component or static text on right side of the date
input component. It accepts Expression
language and/or text values. To include a Button
component as rightAddOn the attribute must contain the same id as the
button specified as inner tag of input component
|
autoFocus | boolean | false | - | Specifies if the date input component must be focused when page is rendered |
tabIndex | integer | false | - | Specifies the tab index for the date input component be focused when page is traversed by tab key |
dateMode | string | false | - | Specifies the date mode for date picker. Possible values are years, months,
days, timeonly
|
hideIcon | boolean | false | false | Specifies if date button on right side of date input should be hidden. In case
true the date picker is opened when input is clicked
|
showWeeks | boolean | false | false | Specifies if date picker will present the date number on left side of calendar |
defaultDate | string | false | - | Specifies the default value for the date component. It accepts Expression language and/or text
values. Date component can be integrated with String, Date util or Date Joda time on WebBean attribute declared via Expression Language |
locale | string | false | - | Specifies the locale to be used on date. Please check the possible locales on https://github.com/moment/moment/tree/develop/locale |
linkWith | string | false | - | Specifies the id of other date components to be able to use them together, so the linked date component can start by having the date greater than the current date declaring the linkWith attribute |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Upload
Why use it?
Upload component can be used to easily find system files and upload it to server via Expression Language to integrate client side with WebBean on server side.
Where to use it?
It must be placed only inside form
components in your JSP page to provide
pretty styled upload component to your page.
What use inside it?
This component support inner components to provide more functionality such as bind events, Ajax request, buttons and tooltip. Have a look in the following components for more details.
How to use it?
To integrate the upload
component with WebBean you must specify the Expression language on attribute
value
pointing to WebBean attribute name which must be exposed via getter and
setter methods.
The attribute on WebBean must be of type
javax.servlet.http.Part
and also this component must have direct parent tag of
form
component with attribute enctype equal to multipart/form-data
How to place button on right and/or left side?
To place inner button
components aside of the upload there are the attributes
leftAddOn
and rightAddOn
, which generally hold text values, but if
they specify the button id the upload will replace the text by the button component.
<sm:form enctype="multipart/form-data">
<sm:upload label="Simple upload file" value="@{uploadBean.filePart}" />
</sm:form>
<sm:form enctype="multipart/form-data">
<sm:upload label="Upload file validate" value="@{uploadBean.filePart}">
<sm:validate maxLength="1000000" text="File cannot exceed 1MB" />
</sm:upload>
</sm:form>
<sm:form enctype="multipart/form-data">
<sm:upload label="Upload file with addons" value="@{uploadBean.filePart}" leftAddOn="File"
rightAddOn="Upload it" />
</sm:form>
<sm:form enctype="multipart/form-data">
<sm:upload label="Upload file with button addon" value="@{uploadBean.filePart}" leftAddOn="File"
rightAddOn="upload-btn-id">
<sm:button id="upload-btn-id" label="Upload button" look="primary" />
</sm:upload>
</sm:form>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the upload component |
style | string | false | - | Specifies the css style to be applied to upload component along with Bootstrap input styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to upload component along with Bootstrap input styles. It accepts Expression language and/or text values |
rest | string | false | - | Specifies the JSON key name or XML tag name for REST requests using Rest component. Check Rest component for more details |
size | string | false | - | Specifies the Bootstrap size for the upload. Possible values are small,
large
|
value | string | false | - | Specifies the file to be uploaded by upload component. It accepts Expression language and/or text
values. The attribute on WebBean must be of type javax.servlet.http.Part and also the upload component
must have direct parent tag of form component with attribute enctype
equal to multipart/form-data
|
readOnly | boolean | false | - | Specifies if the upload component is read only field |
disabled | boolean | false | - | Specifies if the upload component is disabled |
placeholder | string | false | - | Specifies the placeholder to be presented inside the upload component to easy the field understanding. It accepts Expression language and/or text values |
label | string | false | - | Specifies label for the upload component. It accepts Expression language and/or text values |
leftAddOn | string | false | - | It includes an addon button component or static text on left side of the upload
component. It accepts Expression
language and/or text values. To include a Button
component as leftAddOn the attribute must contain the same id as the
button tag specified as inner tag of upload component
|
rightAddOn | string | false | - | It includes an addon button component or static text on right side of the upload
component. It accepts Expression
language and/or text values. To include a Button
component as rightAddOn the attribute must contain the same id as the
button specified as inner tag of upload component
|
autoFocus | boolean | false | - | Specifies if the upload component must be focused when page is rendered |
tabIndex | integer | false | - | Specifies the tab index for the input component be focused when page is traversed by tab key |
onUpload | string | false | - | Specifies a JavaScript function to be called every time a chunk of file stream is sent to server during the request processing. The function may receive parameters such as event(Object), position(Integer), total(Integer) and percent(Integer) in that order. |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Text area
Why use it?
Text area component can be used to easily exchange long text with server via Expression Language to integrate client side with WebBean on server side.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled text area component to your page.
What use inside it?
This component support inner components to provide more functionality such as bind events, Ajax request and tooltip. Have a look in the following components for more details.
How to use it?
To integrate the textarea
component with WebBean you must specify the Expression language on attribute
value
pointing to WebBean attribute name which must be exposed via getter and
setter methods.
<sm:textarea label="Simple text area" />
<sm:textarea label="Integrated text area" value="@{textAreaBean.value}" />
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the text area component |
style | string | false | - | Specifies the css style to be applied to text area component along with Bootstrap text area styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to text area component along with Bootstrap text area styles. It accepts Expression language and/or text values |
rest | string | false | - | Specifies the JSON key name or XML tag name for REST requests using Rest component. Check Rest component for more details |
length | integer | false | - | Specifies maximum length allowed for text content on text area component |
rows | integer | false | - | Specifies the number of rows for the text area component |
cols | integer | false | - | Specifies the number of columns for the text area component |
value | string | false | - | Specifies the value for the text area component. It accepts Expression language and/or text values. |
readOnly | boolean | false | - | Specifies if the text area component is read only field |
disabled | boolean | false | - | Specifies if the text area component is disabled |
placeholder | string | false | - | Specifies the placeholder to be presented inside the text area component to easy the field understanding. It accepts Expression language and/or text values |
label | string | false | - | Specifies label for the text area component. It accepts Expression language and/or text values |
tabIndex | integer | false | - | Specifies the tab index for the text area component be focused when page is traversed by tab key |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Image components
JSmart provide a some image components with useful features and pretty styled elements provided via Bootstrap integration.
Icon
Why use it?
Icon component can be used to easily include glyphicons from Bootstrap or other sources on client page and also integrate client side with WebBean on server side via Expression Language to perform Ajax requests.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled icon component to your page.
What use inside it?
This component support inner components to provide more functionality such as bind events, Ajax request and tooltip. Have a look in the following components for more details.
How to use it?
Include the icon component into your JSP page to provide glyphicons as standalone icon or inside another components for placing icon internally into those components such as the following.
<sm:icon name="glyphicon-globe" />
<sm:icon name="glyphicon-globe" look="primary"/>
<sm:icon name="glyphicon-globe" style="font-size: 24px;" look="danger" />
<sm:link outcome="/components.html#icon" label="Icon link">
<sm:icon name="glyphicon-link" />
</sm:link>
<sm:button label="Button label">
<sm:icon name="glyphicon-user" side="right" />
</sm:button>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the icon component |
style | string | false | - | Specifies the css style to be applied to icon component. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to icon component. It accepts Expression language and/or text values |
name | string | false | - |
Specifies name of Boostrap glyphicon to be print on page. Check the link
|
side | string | false | - | Specifies side on which the icon will be placed. Possible values are right and
left
|
look | string | false | - | Specifies the Bootstrap look for the icon. The possible values are primary,
success, info, warning, danger and default . It accepts Expression language and/or text
values
|
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Image
Why use it?
Image component can be used to easily include internal or external images on client page and also integrate client side with WebBean on server side via Expression Language to perform Ajax requests.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled image component to your page.
What use inside it?
This component support inner components to provide more functionality such as bind events, Ajax request and tooltip. Have a look in the following components for more details.
How to use it?
JSmart follows a convention for the that images must be placed inside webapp
folder and the relative path starting from webapp folder is used as lib
attribute for image component. This path specify folders which can be separated via periods
(.) or slashes (/).
For example, one the image placed on webapp/css/images/my_image.png
you can use the component as following:
<sm:image lib="css.images"
name="my_mage.png" />
For external image references the lib
can be ignore and only name
attribute must be specified.
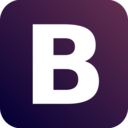
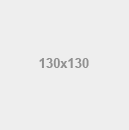
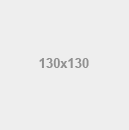
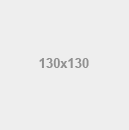
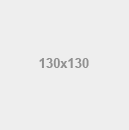
<sm:image name="http://www.w3schools.com/bootstrap/bs.png" />
<sm:image lib="css.images" name="img_example.png" />
<sm:image lib="css.images" name="img_example.png" type="round" />
<sm:image lib="css.images" name="img_example.png" type="circle" />
<sm:image lib="css.images" name="img_example.png" type="thumbnail" />
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the image component |
style | string | false | - | Specifies the css style to be applied to image component. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to image component. It accepts Expression language and/or text values |
name | string | true | - | Specifies relative or static image name to be displayed. In case relative name, the lib attribute must be specified, so the image can be located inside your webapp folder. It accepts Expression language and/or text values |
lib | string | false | - | Specifies the path in which the image is stored inside your webapp folder. It accepts Expression language and/or text values |
width | string | false | - | Specifies width to be applied on image |
height | string | false | - | Specifies the height to be applied on image |
alt | string | false | - | Specifies the alternative name case image cannot be loaded. It accepts Expression language and/or text values |
caption | string | false | - | Specifies caption for the image of type figure. It accepts Expression language and/or text values |
figure | boolean | false | - | Specifies if the image should use the figure HTML element |
type | string | false | - | Specifies the type of the image. Possible values are responsive, round, circle
and thumbnail
|
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Form components
JSmart provide a some form containers for sending a set of data to server side with useful features and pretty styled elements provided via Bootstrap integration.
Form
Why use it?
Form component can be used to easily provide page content submit via Ajax request or Form submit to server side. It also provide nice functionality for field validation and size modification using pretty components provided via Bootstrap integration.
Where to use it?
Include the form component anywhere into your JSP page to provide fields submit via Form
submit or Ajax request.
Just do not place form components inside other form components.
How to use it?
For any Ajax request triggered from form component, all field values inside it will be carried to server side. If a component inside form component specifies Validate component, the field value must be valid otherwise the validation will present a error as configured on component.
<sm:form size="small">
<sm:input label="Input value" value="@{formBean.inputValue}">
<sm:validate look="warning" />
</sm:input>
<sm:select label="Select value" selectValues="@{formBean.selectValues}">
<sm:validate look="success" />
<sm:option label="" value="" />
<sm:option label="Value one" value="value_one" />
<sm:option label="Value two" value="value_two" />
</sm:select>
<sm:checkgroup label="Check group value" selectValues="@{formBean.checkValues}">
<sm:validate look="error" />
<sm:check label="Value one" value="value_one" />
<sm:check label="Value two" value="value_two" />
</sm:checkgroup>
<sm:button id="form-btn" ajax="true" label="Button label" action="@{buttonBean.doAction}">
<sm:load />
</sm:button>
</sm:form>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the form component |
style | string | false | - | Specifies the css style to be applied to form component along with Bootstrap form styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to form component along with Bootstrap form styles. It accepts Expression language and/or text values |
method | string | false | post | Specifies form submit method. Possible values are post and get |
enctype | string | false | - | Specifies form content type to be submitted to server side |
disabled | string | false | - | Specifies if all components inside form are disabled |
position | string | false | - | Specifies the disposition of elements inside the form. Possible values inline
and horizontal . In case horizontal , the labels for internal form
components will be placed horizontally to its respective component. In case inline ,
all fields will have its disposition inline instead of standard column
|
size | string | false | - | Specifies the size of components inside the form. Possible values large and
small
|
Rest
Why use it?
Rest component can be used to easily provide REST capabilities via Ajax request with JSON or XML body content. It also provide nice functionality for field validation and size modification using pretty elements provided via Bootstrap integration.
Where to use it?
Include the rest component into your JSP page to provide fields wrapping into JSON or XML
content for REST request.
Just do not place rest components inside other rest
components.
How to use it?
If a component inside rest component specifies Validate
component, the field value must be valid otherwise the validation will present a error as
configured on component.
All fields intended to be sent as body content must specify
the rest attribute to work as key for JSON or tag name for XML.
For query parameters
request using rest component, include one ore more Param
components inside the component which will start the action, i.e., the Button or Link
components.
<sm:rest size="small" endpoint="/api/v1/test" method="post">
<sm:input label="Input value" rest="inputAttr" value="@{restBean.inputValue}">
<sm:validate look="warning" />
</sm:input>
<sm:select label="Select value" rest="selectAttr" selectValues="@{restBean.selectValues}">
<sm:validate look="success" />
<sm:option label="" value="" />
<sm:option label="Value one" value="value_one" />
<sm:option label="Value two" value="value_two" />
</sm:select>
<sm:checkgroup label="Check group value" rest="checkgroupAttr" selectValues="@{restBean.checkValues}">
<sm:validate look="error" />
<sm:check label="Value one" value="value_one" />
<sm:check label="Value two" value="value_two" />
</sm:checkgroup>
<sm:button id="rest-btn" ajax="true" label="Button label" action="@{restBean.doAction}">
<sm:load />
<sm:param name="queryParamOne" value="Value one" />
<sm:param name="queryParamTwo" value="Value two" />
</sm:button>
</sm:rest>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the rest component |
style | string | false | - | Specifies the css style to be applied to rest component. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to rest component. It accepts Expression language and/or text values |
endpoint | string | true | - | Specifies the endpoint to be accessed via REST request. It can be relative or static URL |
method | string | true | - | Specifies the HTTP method to be used for REST request. Possible values are post,
get, put, delete, patch, head and options
|
contentType | string | false | - | Specifies content type for body content creation to send via REST request. Possible
values are json and xml
|
cors | boolean | false | - | Specifies if REST request is performed via cross origin. If true the request will specify the Ajax jsonp as true. For more details about jsonp attribute check the link http://api.jquery.com/jquery.ajax |
jsonpCallback | string | false | - | Specifies the query parameter to be sent along REST request using jsonp attribute for Ajax request. For more details about jsonpCallback attribute check the link http://api.jquery.com/jquery.ajax |
bodyRoot | string | false | - | Specifies body content root node. For JSON content-type it refers to root node to wrap the content. For XML content-type it refers to xml root tag |
position | string | false | - | Specifies the disposition of component inside the rest. Possible values inline
and horizontal
|
size | string | false | - | Specifies the size of elements inside the rest. Possible values large and
small
|
Validate components
JSmart provide a some validate components for client and/or server side validation process with useful features and pretty styled elements provided via Bootstrap integration.
Validate
Why use it?
Validate component can be used to easily provide input components validation before the Form is submitted or the Ajax request is performed.
Where to use it?
It is meant for usage only inside input components, but the validation only apply if those
input components are placed inside a form
or rest
component
regardless regular submit or Ajax request. The following components accept the validation
component inside it.
How to use it?
The input components must wrap the validate
tag inside it and for the
validation to apply those input components must be placed inside form
or rest
component.
For upload
component the minLength
maxLength
applies for file size measured in bytes.
For regex
attribute it uses
JavaScript RegExp function.
For making the input component required only place the
validate component inside it.
<sm:form>
<sm:input label="Input with minimum length">
<sm:validate minLength="3" text="Length must be greater than 3 characters" />
</sm:input>
<sm:input label="Input with maximum length">
<sm:validate maxLength="8" text="Length must be less than 8 characters" look="warning" />
</sm:input>
<sm:input label="Input with regex">
<sm:validate regex=".*@.*" text="Input must contain at(@)" look="success" />
</sm:input>
<sm:checkbox label="Checkbox required">
<sm:validate text="Checkbox check is required" look="error" />
</sm:checkbox>
<sm:upload label="File upload maximum size">
<sm:validate maxLength="1000000" text="File cannot be greater than 1MB" />
</sm:upload>
<sm:radiogroup label="Radio group check required" inline="true">
<sm:check label="Label one" value="value_one" />
<sm:check label="Label two" value="value_two" />
<sm:check label="Label three" value="value_three" />
<sm:validate text="Radio group check is required" look="warning" />
</sm:radiogroup>
<sm:checkgroup label="Check group required" inline="true">
<sm:check label="Label one" value="value_one" />
<sm:check label="Label two" value="value_two" />
<sm:check label="Label three" value="value_three" />
<sm:validate text="Check group check is required" look="success" />
</sm:checkgroup>
<sm:select label="Select required">
<sm:option label="" value="" />
<sm:option label="Label one" value="value one" />
<sm:option label="Label two" value="value two" />
<sm:option label="Label three" value="value three" />
<sm:validate text="Selection is required" look="error" />
</sm:select>
<sm:date label="Date required">
<sm:validate text="Date input is required" look="warning" />
</sm:date>
<sm:textarea label="Text area required">
<sm:validate text="Text are is required" look="success" />
</sm:textarea>
<sm:button label="Validate components" action="" />
</sm:form>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
text | string | false | - | Specifies the text to presented when validation fails. It accepts Expression language and/or text values. |
look | string | false | - | Specifies the look to change the input component when validation fails. Possible
values are success, error, warning . It accepts Expression language and/or text
values.
|
minLength | integer | false | - | Specifies the minimum length to consider the input component valid. For upload
component it means minimum number of file bytes.
|
maxLength | integer | false | - | Specifies the maximum length to consider the input component valid. For upload
component it means maximum number of file bytes.
|
regex | string | false | - | Specifies the regex to be applied on input text value to consider it valid. The regex use RegExp function from JavaScript for validation process. |
Authorize
Why use it?
Authorize component can be used for partial page rendering depending on login roles provided by AuthenticateBean implementation.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide controlled rendering depending on login roles.
How to use it?
The authorize
component must be used in conjunction with the following
components.
<sm:authorize>
<when grant="role_one, role_two, role_three" deny="role_four">
Any tag or component declaration to be rendered when login roles contains one of the
grant roles (role_one, role_two or role_three) and do not contain the deny role (role_four)
</when>
<otherwise>
Any tag or component declaration to be rendered case login roles do no fit on
the above conditions
</otherwise>
</sm:authorize>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
- | - | - | - | - |
When
Why use it?
When component can be used to easily provide partial page rendering based on declared login roles specified via AuthenticateBean implementation.
Where to use it?
This component is meant to be used internally to authorize
component to provide
the list of grant or deny roles allowed to see this tag content.
How to use it?
The when
component must declare the grant
or deny
attribute containing a list of roles separated by comma (,) to be used to check against
login roles provided by attribute annotated with AuthorizeAccess
on AuthenticateBean implementation.
<sm:authorize>
<when grant="role_one, role_two, role_three" deny="role_four" >
Any tag or component declaration to be rendered when login roles contains one of the
grant roles (role_one, role_two or role_three) but do not contain the deny role (role_four)
</when>
<when grant="role_five">
Any tag or component declaration to be rendered when login roles contains the
grant role (role_five)
</when>
<otherwise>
Any tag or component declaration to be rendered case login roles do no fit on
the above conditions
</otherwise>
</sm:authorize>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
grant | string | false | - | Comma separated grant roles to allow when tag content rendering when
the logged user has at least one of the roles declared in this attribute. The list of roles are compared with the List Collection provided by AuthenticateBean via attribute annotated with AuthorizeAccess. It accepts Expression language and/or text values. |
deny | string | false | - | Comma separated deny roles to block when tag content from rendering
when the logged user has all denied roles declared in this attribute. Keep in mind
that the deny roles have precedence over grant declared roles. The list of roles are compared with the List Collection provided by AuthenticateBean via attribute annotated with AuthorizeAccess. It accepts Expression language and/or text values. |
Otherwise
Why use it?
Otherwise component can be used to easily provide partial page rendering in case there is no valid declared login roles specified via AuthenticateBean implementation.
Where to use it?
This component is meant to be used internally to authorize
component to render
its content in case none of the when
conditions match.
How to use it?
The otherwise
component is automatic processed considering the
grant
and deny
roles declared on when
component in
the same hierarchy.
<sm:authorize>
<when grant="role_one, role_two, role_three" deny="role_four">
Any tag or component declaration to be rendered when login roles contains one of the
grant roles (role_one, role_two or role_three) and do not contain deny role (role_four)
</when>
<otherwise>
Any tag or component declaration to be rendered case login roles do no fit on
the above conditions
</otherwise>
</sm:authorize>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
- | - | - | - | - |
ReCaptcha
Why use it?
Integrated with Google ReCaptcha, this component can be used to easily validate on server and client sides if the requester accessing your JSP page is a real person or a robot.
Where to use it?
It can be placed anywhere in your JSP page but it must have direct parent tag of type form
or rest
component.
How to use it?
This component requires a direct form
or rest
component parent
which must contain only one recaptcha
component inside it.
In addition to
provide a validation you must log into Google
Recaptcha and register your site, so you can receive the siteKey
and
secretKey
for validating your captcha on client and server respectively.
The siteKey must be used as recaptcha
attribute while the secretKey must be
used on server side to validate the request via WebContext.
There are two versions of ReCaptcha available for usage the version 1
which presents a custom input aside with image or audio for validation, or the version
2
which is the recent smart implementation from Google to recognize more
effectively if the requester is a robot.
<sm:form>
<sm:recaptcha id="recaptcha-v1" label="ReCaptcha version 1" audioLabel="ReCaptcha audio label"
siteKey="6LfPxwMTAAAAAFKMi9ApdkZhxFFcvstI_shXaafG" placeholder="Enter the captcha here">
</sm:recaptcha>
</sm:form>
<sm:form>
<sm:recaptcha id="recaptcha-v2" label="ReCaptcha version 2" version="2"
locale="en" siteKey="6LfPxwMTAAAAAFKMi9ApdkZhxFFcvstI_shXaafG" />
</sm:form>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the recaptcha component |
style | string | false | - | Specifies the css style to be applied to recaptcha v1 input along with Bootstrap input styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to recaptcha v1 input along with Bootstrap input styles. It accepts Expression language and/or text values |
version | integer | false | 1 | Specifies the version for the Google
ReCaptcha to be used. Possible values are 1, 2
|
siteKey | string | true | - | Specifies the siteKey provided from Google ReCaptcha when your page is manually registered. |
autoFocus | boolean | false | - | Specifies if the recaptcha v1 input must be focused when page is rendered |
placeholder | string | false | - | Specifies the placeholder to be presented inside the recaptcha v1 input component to easy the field understanding. It accepts Expression language and/or text values |
label | string | false | - | Specifies label for the recaptcha component. It accepts Expression language and/or text values |
audioLabel | string | false | - | Specifies label for the recaptcha v1 component when audio is selected for validation. It accepts Expression language and/or text values |
locale | string | false | - | Specifies locale for the recaptcha component. Please have a look on https://developers.google.com/recaptcha/docs/language for possible locales to be used on ReCaptcha version 2. And on https://developers.google.com/recaptcha/old/docs/customization#i18n for possible locales to be used on ReCaptcha version 1. |
disabled | boolean | false | - | Specifies if the recaptcha v1 input is disabled |
tabIndex | integer | false | - | Specifies the tab index for the recaptcha v1 input be focused when page is traversed by tab key |
size | string | false | - | Specifies the Bootstrap size for the recaptcha v1 input. Possible values are small,
large
|
align | string | false | - | Specifies the recaptcha v1 component align. Possible values are left, center,
right
|
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Action components
JSmart provide a series of action components for sending and retrieving content to and from server side with useful features and pretty styled elements provided via Bootstrap integration.
Button
Why use it?
Button component can be used to easily provide action via Ajax request or Form submit to server side via Expression Language to integrate with WebBean.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled button component to your page.
The action, update and callbacks which will be triggered by this component can also can be declared via Annotation on WebBean, have a look at Action on WebBean for more details and examples.
What use inside it?
This component support inner components to provide more functionality such as bind events, Ajax request, parameters, arguments and tooltip. Have a look in the following components for more details.
How to use it?
To integrate the button
component with WebBean you must specify the Expression language on attribute
action
pointing to WebBean method name to process the action when request is
performed.
For Ajax requests, the attribute ajax
must be se to true and
for server response to take effect on client side use the update
for updating
elements on page via id or execute callbacks by informing JavaScript functions to attributes
beforeSend, onSuccess, onError, onComplete
.
<sm:button label="Button label" size="xsmall" />
<sm:button label="Button label" size="small" />
<sm:button label="Button label" look="info" />
<sm:button label="Button label" look="danger">
<sm:icon name="glyphicon-user" />
</sm:button>
<sm:button label="Load button" look="success" ellipsize="true" length="8">
<sm:icon name="glyphicon-start" />
</sm:button>
<sm:button label="Popover button" look="warning">
<sm:popover title="Popover title" content="Popover content" side="right" event="hover" />
</sm:button>
<sm:button label="Form button" look="primary" action="@{buttonBean.doAction}">
<sm:icon name="glyphicon-fire" side="right" />
<sm:load />
</sm:button>
<sm:button id="btn-ajax-1" ajax="true" label="Ajax button" action="@{buttonBean.doActionWithArg}"
onSuccess="alert('On button action success');">
<sm:arg value="argValue" />
<sm:icon name="glyphicon-fire" side="right" />
<sm:load />
</sm:button>
<sm:button id="btn-ajax-2" ajax="true" label="Ajax button" action="@{buttonBean.doAction}">
<sm:icon name="glyphicon-fire" />
<sm:load />
<sm:dropmenu segmented="true">
<sm:dropaction action="@{buttonBean.doActionOne}" label="Action one">
<sm:arg value="argValueOne" />
</sm:dropaction>
<sm:dropaction action="@{buttonBean.doActionTwo}" label="Action two">
<sm:param name="@{buttonBean.paramOne}" value="paramValue" />
</sm:dropaction>
</sm:dropmenu>
</sm:button>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the button component |
style | string | false | - | Specifies the css style to be applied to button component along with Bootstrap btn styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to button component along with Bootstrap btn styles. It accepts Expression language and/or text values |
label | string | false | - | Specifies the label for the button. It accepts Expression language and/or text values |
length | integer | false | - | Specifies the maximum length before trimming the button label |
ellipsize | boolean | false | - | Specifies if the button label will receive the ... characters at the
end of text after is has been trimmed
|
size | string | false | - | Specifies Bootstrap size for the button. Possible values are xsmall, small,
large and justified
|
look | string | false | - | Specifies the Bootstrap look for the button. The possible values are primary,
success, info, warning, danger, default . It accepts Expression language and/or text
values
|
ajax | boolean | false | - | Specifies if the action should use Ajax request instead of Form submit |
onForm | string | false | - | Used only when ajax attribute is true in order to specify which form to use for Ajax Request to send the form content. If button is already inside a form this attribute is ignored during request processing |
reset | boolean | false | - | Specifies if the button is meant to be used as reset input to reset other inputs in the same Form HTML element |
disabled | boolean | false | - | Specifies if the button is disabled |
tabIndex | integer | false | - | Specifies the tab index for the HTML element to be traversed when tab key is pressed |
action | string | false | - | Specifies the action to be executed on server side via Ajax request or Form submit. It must use Expression language to specify the method to be executed on WebBean |
update | string | false | - | Specifies id elements to be updated after Ajax request completes. The ids must be specified as comma separated values so the HTML elements can be update with request response content. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the Ajax request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Button group
Why use it?
Button group component can be used to easily provide group of actions via Ajax request or Form submit to server side via Expression Language to integrate with WebBean.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled button components to your page.
What use inside it?
This component support only button components inside it. Have a look in the following component for more details.
<sm:buttongroup>
<sm:button label="Button one" />
<sm:button label="Button two" look="warning">
<sm:icon name="glyphicon-fire" />
</sm:button>
<sm:button label="Button three" look="danger">
<sm:dropmenu segmented="true">
<sm:dropaction label="Action One" />
</sm:dropmenu>
</sm:button>
</sm:buttongroup>
<sm:buttongroup inline="false">
<sm:button label="Button one" />
<sm:button label="Button two" look="primary">
<sm:icon name="glyphicon-fire" />
</sm:button>
<sm:button label="Button three" look="success">
<sm:dropmenu>
<sm:dropaction label="Action One" />
</sm:dropmenu>
</sm:button>
</sm:buttongroup>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the buttongroup component |
style | string | false | - | Specifies the css style to be applied to buttongroup component along with Bootstrap btn-group styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to buttongroup component along with Bootstrap btn-group styles. It accepts Expression language and/or text values |
inline | boolean | false | true | Specifies if the button group will be presented inline or in column |
Link
Why use it?
Button component can be used to easily provide page redirection or action via Ajax request to server side via Expression Language to integrate with WebBean.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled link component to your page.
The action, update and callbacks which will be triggered by this component can also can be declared via Annotation on WebBean, have a look at Action on WebBean for more details and examples.
What use inside it?
This component support inner components to provide more functionality such as bind events, Ajax request, parameters, arguments and tooltip. Have a look in the following components for more details.
How to use it?
To use link
as regular link element you must specify the outcome
attribute, otherwise to integrate the link
component with WebBean you must specify the Expression language on attribute action
pointing to WebBean method name to process the action when request is performed.
In
case action attribute is specified only Ajax request is performed and for the server
response to take effect on client side use the update
for updating elements on
page via id or execute callbacks by informing JavaScript functions to attributes beforeSend,
onSuccess, onError, onComplete
.
<sm:link outcome="/components.html#link" />
<sm:link outcome="/components.html#link" label="Link redirect">
<sm:icon name="glyphicon-link" />
</sm:link>
<sm:link label="Link action" action="@{linkBean.doAction}">
<sm:icon name="glyphicon-link" />
<sm:load />
</sm:link>
<sm:link label="Link dropdown">
<sm:icon name="glyphicon-star" />
<sm:dropmenu>
<sm:dropaction label="Action one" />
<sm:dropaction label="Action two" />
</sm:dropmenu>
</sm:link>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the link component |
style | string | false | - | Specifies the css style to be applied to link component along with Bootstrap btn-link styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to link component along with Bootstrap btn-link styles. It accepts Expression language and/or text values |
label | string | false | - | Specifies the label for the link. It accepts Expression language and/or text values |
length | integer | false | - | Specifies the maximum length before trimming the link label |
ellipsize | boolean | false | - | Specifies if the link label will receive the ... characters at the end
of text after is has been trimmed
|
size | string | false | - | Specifies Bootstrap size for the link. Possible values are xsmall, small,
large and justified
|
target | string | false | - | Specifies the target for the link component. |
look | string | false | - | Specifies the Bootstrap look for the link. The possible values are primary,
success, info, warning, danger and default . It accepts Expression language and/or text
values
|
onForm | string | false | - | Used along with action attribute in order to specify which form to use for Ajax Request to send the form content. If link is already inside a form this attribute is ignored during request processing |
outcome | string | false | - | Specifies the internal or external URL to redirect the page. When this value is specified the action attribute will not take effect |
disabled | boolean | false | - | Specifies if the link is disabled |
tabIndex | integer | false | - | Specifies the tab index for the HTML element to be traversed when tab key is pressed |
action | string | false | - |
Specifies the action to be executed on server side via Ajax request. It must use Expression language to specify the
method to be executed on
|
update | string | false | - | Specifies id elements to be updated after request completes. The ids must be specified as comma separated values so the HTML elements can be update with request response content. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the Ajax request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Dropdown
Why use it?
Dropdown component can be used to easily provide dropdown list of actions via Ajax request to server side via Expression Language to integrate with WebBean.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled dropdown component to your page.
What use inside it?
This component support inner components to provide more functionality such as bind events, Ajax request, icons and drop menu. Have a look in the following components for more details.
How to use it?
Drop down is meant to be used along with Drop menu to provide a list of actions using Ajax request to be performed on server side via Expression Language integration.
<sm:dropdown label="Dropdown label">
<sm:icon name="glyphicon-fire" />
</sm:dropdown>
<sm:dropdown label="Dropdown label">
<sm:icon name="glyphicon-fire" side="right" />
</sm:dropdown>
<sm:dropdown label="Dropdown label">
<sm:dropmenu>
<!-- Add dropaction for item action -->
</sm:dropmenu>
</sm:dropdown>
<sm:dropdown label="Dropdown label">
<sm:icon name="glyphicon-fire" />
<sm:dropmenu>
<sm:dropaction label="Action one" />
</sm:dropmenu>
</sm:dropdown>
<sm:dropdown label="Dropdown label">
<sm:dropmenu>
<sm:dropaction label="Action one" />
<sm:dropaction label="Action two" divider="true" />
<sm:dropaction label="Action three" />
</sm:dropmenu>
</sm:dropdown>
<nav class="navbar navbar-default">
<div class="container-fluid">
<ul class="nav navbar-nav">
<sm:dropdown label="Dropdown one" navbar="true">
<sm:icon name="glyphicon-fire" />
<sm:dropmenu>
<sm:dropaction label="Action one" />
<sm:dropaction label="Action two" divider="true" />
<sm:dropaction label="Action three" />
</sm:dropmenu>
</sm:dropdown>
<sm:dropdown label="Dropdown two" navbar="true">
<sm:icon name="glyphicon-flag" />
<sm:dropmenu>
<sm:dropaction label="Action one" />
<sm:dropaction label="Action two" />
<sm:dropaction label="Action three" divider="true" />
<sm:dropaction label="Action four" />
</sm:dropmenu>
</sm:dropdown>
</ul>
</div>
</nav>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the dropdown component |
style | string | false | - | Specifies the css style to be applied to dropdown component along with Bootstrap dropdown styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to dropdown component along with Bootstrap dropdown styles. It accepts Expression language and/or text values |
label | string | true | - | Specifies the label for the dropdown. It accepts Expression language and/or text values |
navbar | boolean | false | false | Specifies if this dropdown belongs to a nav bar HTML element. It will differentiate if the generated HTML for this dropdown will use div or li HTML element as wrapper. |
disabled | boolean | false | false | Specifies if this dropdown is disabled |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Drop menu
Why use it?
Drop menu component can be used to easily provide menu of actions using Ajax request to server side via Expression Language to integrate with WebBean.
Where to use it?
It is meant to be used inside button
, link
or
dropdown
components to provide menu of actions executed via Ajax request to
server side.
<sm:dropdown label="Dropdown label">
<sm:dropmenu>
<sm:dropaction label="Action one" />
</sm:dropmenu>
</sm:dropdown>
<sm:link label="Link label">
<sm:dropmenu>
<sm:dropaction label="Action one" />
</sm:dropmenu>
</sm:link>
<sm:button label="Button label">
<sm:dropmenu>
<sm:dropaction label="Action one" />
</sm:dropmenu>
</sm:button>
<sm:button label="Button label">
<sm:dropmenu dropUp="true">
<sm:dropaction label="Action one" />
</sm:dropmenu>
</sm:button>
<sm:button label="Button label">
<sm:dropmenu segmented="true">
<sm:dropaction label="Action one" />
</sm:dropmenu>
</sm:button>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the dropmenu component |
style | string | false | - | Specifies the css style to be applied to dropmenu component along with Bootstrap dropmenu styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to dropmenu component along with Bootstrap dropmenu styles. It accepts Expression language and/or text values |
align | string | false | - | Specifies the alignment for the drop menu related to its wrapper. Possible values
are left or right
|
segmented | boolean | false | false | Specifies if drop menu caret is segmented from its wrapper. It is only applied for Button wrapper tag |
dropUp | boolean | false | false | Specifies if the drop menu will be opened from top to bottom or from bottom to top |
Drop action
Why use it?
Drop action component can be used to easily provide drop menu item action using Ajax request to server side via Expression Language to integrate with WebBean.
Where to use it?
It is meant to be used inside Drop menu components to provide menu item actions executed via Ajax request to server side.
The action, update and callbacks which will be triggered by this component can also can be declared via Annotation on WebBean, have a look at Action on WebBean for more details and examples.
What use inside it?
This component support inner components to provide more functionality such as parameters, arguments and icons. Have a look in the following components for more details.
<sm:dropdown label="Dropdown label">
<sm:dropmenu>
<sm:dropaction label="Action one" />
</sm:dropmenu>
</sm:dropdown>
<sm:dropdown label="Dropdown label">
<sm:dropmenu>
<sm:dropaction label="Action one" divider="true">
<sm:icon name="glyphicon-fire" />
</sm:dropaction>
<sm:dropaction label="Action two">
<sm:icon name="glyphicon-fire" side="right" />
</sm:dropaction>
</sm:dropmenu>
</sm:dropdown>
<sm:dropdown label="Dropdown label">
<sm:dropmenu>
<sm:dropaction header="Action header" label="Action one" />
<sm:dropaction label="Action two" divider="true" />
<sm:dropaction header="Action header" label="Action three" />
</sm:dropmenu>
</sm:dropdown>
<sm:dropdown label="Dropdown label">
<sm:dropmenu>
<sm:dropaction header="Action header" label="Action one" divider="true"
action="@{dropActionBean.doActionOne}"
onSuccess="alert('On action one success');" />
<sm:dropaction header="Action header" label="Action two"
action="@{dropActionBean.doActionTwo}"
onComplete="alert('On action two complete');" />
</sm:dropmenu>
</sm:dropdown>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the dropaction component |
style | string | false | - | Specifies the css style to be applied to dropaction component along with Bootstrap dropaction styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to dropaction component along with Bootstrap dropaction styles. It accepts Expression language and/or text values |
header | string | false | - | Specifies the header for the dropaction. It accepts Expression language and/or text values |
label | string | false | - | Specifies the label for the dropaction. It accepts Expression language and/or text values |
divider | boolean | false | false | Specifies if this dropaction will present a divider at the bottom side |
disabled | boolean | false | false | Specifies if this dropaction is disabled |
outcome | string | false | - | Specifies the internal or external URL to redirect the page. When this value is specified the action attribute will not take effect |
action | string | false | - |
Specifies the action to be executed on server side via Ajax request. It must use Expression language to specify the
method to be executed on
|
update | string | false | - | Specifies id elements to be updated after request completes. The ids must be specified as comma separated values so the HTML elements can be update with request response content. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Ajax
Why use it?
Ajax component can provide action via Ajax request to server side using Expression Language for integration with WebBean.
Where to use it?
It can only be used inside the following components to provide Ajax request to server triggered by event on wrapper component.
- Autocomplete
- Badge
- Button
- Checkbox
- Date
- Dropdown
- Footer
- Header
- Icon
- Image
- Input
- Label
- Load
- Output
- Progressbar
- Progress group
- Recaptcha
- Row
- Select
- Table
- Text area
- Upload
The action, update and callbacks which will be triggered by the wrapping component over ajax can also can be declared via Annotation on WebBean, have a look at Action on WebBean for more details and examples.
What use inside it?
This component support some inner components to provide more functionality such as request parameters and/or arguments. Have a look in the following components for more details.
How to use it?
To integrate the ajax
component with WebBean
you must specify the Expression language on
attribute action
pointing to WebBean method name to process the action when
Ajax request is performed.
In addition for server response take effect on client side
use the update
attribute for updating elements on page via their ids or execute
callbacks by informing JavaScript functions to attributes beforeSend, onSuccess,
onError, onComplete
.
<sm:input value="@{inputBean.inputVal}">
<ajax event="blur" action="@{inputBean.action}" beforeSend="myBeforeRequestFn"
update="element-id-one, element-id-two" onSuccess="mySuccessFn" onError="myErrorFn"
onComplete="myCompleteFn">
<!-- Used to set argument value on method called action on WebBean -->
<arg name="argOne" bindTo="other-input-id" />
<!-- Used to set attribute value on WebBean when Ajax request is executed -->
<param name="@{inputBean.otherAttr}" value="Any value" />
</ajax>
</sm:input>
<!-- JavaScript -->
<script type="text/javascript">
function myBeforeRequestFn(jqXHR, settings) {
// Called before Ajax request is executed
}
function mySuccessFn(data, textStatus, jqXHR) {
// Called when Ajax request succeed
}
function myErrorFn(jqXHR, textStatus, error) {
// Called when Ajax request fails
}
function myCompleteFn(jqXHR, textStatus) {
// Called when Ajax request completes
}
</script>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
event | string | true | - | Specifies the event triggered on wrapper component to be used to initiate the ajax
request. Possible values are select, change, blur, click, dbclick, mousedown,
mousemove, mouseover, mouseout, mouseup, keydown, keypress, keyup, focus,
submit
|
onForm | string | false | - | Used specify which form to use for Ajax Request to send the form content. If component containing ajax tag is already inside a form this attribute is ignored during request processing |
action | string | true | - | Specifies the action to be executed on server side via Ajax request. It must use Expression language to specify the method to be executed on WebBean |
update | string | false | - | Specifies id elements to be updated after Ajax request completes. The ids must be specified as comma separated values so the HTML elements can be update with request response content. |
timeout | integer | false | - | Specifies the timeout in milliseconds to wait before Ajax request is performed. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the Ajax request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
Ajax attach
Why use it?
Ajax component can guarantee that any Ajax request triggered from current page will carry
the attached content specified as inner tags of ajaxattach
tag.
Where to use it?
It can be placed anywhere in your JSP page but must remain outside any other component
especially form
component.
What use inside it?
This components only support input
components as inner tag which must specify
their values following the Expression language
to point to any setter methods on bean for setting its values when any Ajax request is
triggered from page.
<sm:ajaxattach id="ajax-fixed-content">
<sm:input id="hidden-value-one" type="hidden" value="@{ajaxBean.valueOne}" />
<sm:input id="hidden-value-two" type="hidden" value="@{ajaxBean.valueTwo}" />
<sm:input id="hidden-value-three" type="hidden" value="@{ajaxBean.valueThree}" />
</sm:ajaxattach>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the ajaxattach component |
style | string | false | - | Specifies the css style to be applied to ajaxattach component. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to ajaxattach component. It accepts Expression language and/or text values |
Param
Why use it?
Param component is meant to be used inside ajax
, function
, text
or output
components to provide parameter values for Ajax request or output
texts.
Where to use it?
It can only be used inside the following components to provide parameters along with the action on server side or to provide parameters for text formatting.
How to use it?
The parameters declared inside the ajax
or function
components
must be linked to WebBean attribute exposed
via getter and setter.
For parameters declared inside the text
or output
components they will be used to replace the %s
or {number}
characters on the result text value. Check those components for more details
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
name | string | true | - | Specifies the name of the WebBean attribute exposed via getter and setter to be used to set the parameter value when Ajax request is made to server. It accepts Expression language and/or text values |
value | string | true | - | Specify the value to be sent to the WebBean attribute exposed via getter and setter when Ajax request is made to server. It accepts Expression language and/or text values |
Arg
Why use it?
Arg component is meant to be used inside ajax
or function
components to provide arguments for action methods on server or function arguments on
client.
Where to use it?
It can only be used inside the following components to provide arguments for action on server side mapped via WebBean method with arguments or to provide arguments for JavaScript functions on client side.
How to use it?
The order of arguments declared inside the ajax
or function
components are important and will be used to assign values to WebBean method or JavaScript function.
In addition the arg
component supports the bindTo
attribute
to retrieve the argument value from the indicated input before the Ajax request is done or
function is called.
Check those components for more details
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
name | string | false | - | Specifies the name of the argument which must be unique among the arguments. It accepts Expression language and/or text values |
value | string | false | - | Specify the value for the argument, case bindTo attribute is specified
the value is ignored. It accepts Expression
language and/or text values
|
bindTo | string | false | - | Specify any input id to be used to retrieve the value before the Ajax request is done or JavaScript function is called. It accepts Expression language and/or text values |
Bind
Why use it?
Bind component can be used to execute JavaScript function on client side based on triggered events on component wrapping the bind component.
Where to use it?
It can only be used inside the following components to execute JavaScript functions on client triggered by event on wrapper component.
<sm:input value="@{inputBean.inputVal}">
<bind event="focus" execute="myFunction" />
</sm:input>
<!-- JavaScript -->
<script type="text/javascript">
function myFunction() {
// Handle event
}
</script>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
event | string | true | - | Specifies the event triggered on wrapper component to be used to call the
JavaScript functions specified on execute attribute. Possible values
are select, change, blur, click, dbclick, mousedown, mousemove, mouseover,
mouseout, mouseup, keydown, keypress, keyup, focus, submit
|
execute | integer | true | - | Specifies the JavaScript functions to be executed when event is triggered on wrapper component. |
timeout | integer | false | - | Specifies the timeout in milliseconds to wait before JavaScript functions are called. |
Function
Why use it?
Function component provides an easy way to map JavaScript function to trigger action on server side via Ajax request.
Where to use it?
It is a standalone component and can be declared anywhere in your JSP page.
The functions also can be declared via Annotation on WebBean, have a look at Function on WebBean for more details and examples.
What use inside it?
This component supports arg
component which is used to specify JavaScript
function arguments to be forwarded to WebBean
which must contain the same sequence of arguments. Also this component support
param
component used to set server values on WebBean attribute exposed via getter and
setter when Ajax request is performed. Have a look in the following component for more
details.
How to use it?
To integrate the function
component with WebBean you must specify the Expression language on attribute
action
pointing to WebBean method name to process the action when Ajax request
is performed.
In addition for server response take effect on client side use the update
attribute for updating elements on page via their ids or execute callbacks by informing
JavaScript functions to attributes beforeSend, onSuccess, onError, onComplete
.
This function is exposed with the name defined on name
attribute and must
be called programmatically following the same sequence of arg
specified as
internal components.
<sm:function id="my-func" name="myFunction" action="@{funcBean.action}">
<sm:arg name="argOne" />
<sm:arg name="argTwo" bindTo="input-id" />
<sm:param name="@{funcBean.attrName}" value="Attr value" />
</sm:function>
<!-- JavaScript -->
<script type="text/javascript">
function myRoutine() {
// Call mapped function to trigger action on server side
myFunction('Value one', 'Value two');
}
</script>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id to link the component to the function exposed via JavaScript |
name | string | true | - | Specifies the name of the function exposed via JavaScript to be manually called to trigger the action to be executed on server side via Ajax request. The name must be compatible with JavaScript function names. |
action | string | true | - | Specifies the action to be executed on server side via Ajax request. It must use Expression language to specify the method to be executed on WebBean |
update | string | false | - | Specifies id elements to be updated after Ajax request completes. The ids must be specified as comma separated values so the HTML elements can be update with request response content. |
timeout | integer | false | - | Specifies the timeout in milliseconds to wait before Ajax request is performed. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the Ajax request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
Async
Why use it?
Async component provides an easy way to receive Server Sent Events on JavaScript callback functions.
Where to use it?
It is a standalone component and can be declared anywhere in your JSP page.
What use inside it?
This component only supports the asyncevent
component inside it to specify the
event name to be linked on server for receiving server events. Have a look in the following
component for more details.
How to use it?
To integrate the async
component with AsyncBean you must specify the the same
path
attribute value as defined on asynchronous bean.
In addition it is
needed to specify at least one asyncevent
including the event name which should
be used to send events via WebContext
writeResponseAsEventStream method.
<sm:async id="async-id" path="/myUrl/event" onStart="onEventStart">
<sm:asyncevent event="my-event" execute="updateEvent" />
</sm:async>
<!-- Callback functions -->
<script type="text/javascript">
function updateEvent(event) {
// Do whatever with event.data
}
function onEventStart(source, id) {
// Save source for later source.close();
}
</script>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the async component |
path | string | true | - | Specifies the relative URL path to start the request to receive async events from server (Server Sent Events - SSE) based on AsyncBean mapped on server side |
withCredentials | boolean | false | false | Must be true when Server Sent Events - SSE are requested from
different origin (Cross Origin Request Security - CORS) but the server side must
return the header "Access-Control-Allow-Origin: *".
|
withCredentials | boolean | false | false | Must be true when Server Sent Events - SSE are requested from
different origin (Cross Origin Request Security - CORS) but the server side must
return the header "Access-Control-Allow-Origin: *".
|
onStart | string | false | - | Specifies the JavaScript callback function to be called when Server Sent Events start receiving content from server. The callback may receive source (EventSource Object) and id (String) as function arguments. |
Async Event
Why use it?
Async event component is meant to be used as inner tag of async
component to
provide an event name to link with JavaScript callback function for every server sent event.
Where to use it?
The asyncevent
component only takes effect inside the Async component. Check some examples on this component
section.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
event | string | true | - | Specifies the event name to be bound for receiving async data from server |
execute | string | true | - | Specifies the JavaScript function to be called every server event sent to the
client. The function may receive the argument event (Event Object) containing the
data with custom object sent.
|
capture | boolean | false | false | Indicates that all events of the specified type will be dispatched to the callback
function specified on execute before being dispatched to any
EventTarget beneath it in the DOM tree.
|
Dynamic scroll components
JSmart provide some of dynamic content components for bringing small chunks of content dynamically from server with useful features and pretty styled elements provided via Bootstrap integration.
Autocomplete
Why use it?
Autocomplete component is a elegant solution for searching values on server based on partial input and provide a dynamic scrolling list of options for selection. It also integrates with server side via Expression Language to WebBean.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled autocomplete component to your page.
What use inside it?
The main and fundamental component to be used inside autocomplete is the Row component to provide a template for creating a list of options from the result of search based on partial input. But this component also support other inner components to provide more functionality such as bind events, load, Ajax request, buttons, icons and tooltip. Have a look in the following components for more details.
How to use it?
To integrate the autocomplete
component with WebBean you must specify the Expression language on attribute
value
pointing to WebBean attribute name which must be exposed via getter and
setter methods and also the attribute values
which must point to List
Collection or to ListAdapter to provide dynamic
scrolling content when searching results.
Some other attributes are required such as
scrollSize
and maxHeight
when using ListAdapter to search for results.
Also the
var
attribute must be provided to be used while iterating to create a list with
searched results. In addition the provider for the list of values only needs to be exposed
via getter method.
Another peculiarities include the inputText
that if
informed will be used to present the selection result on input and the value
attribute that will be sent to server via separated attribute on WebBean which must be
exposed via getter and setter methods.
<sm:autocomplete id="autocptl-0" var="obj" value="@{autoBean.inputVal}" values="@{autoBean.listValues}"
label="Autocomplete using static list" placeholder="Enter the word here for searching results"
inputText="@{obj.title}">
<sm:row>
<sm:header title="@{obj.title}" />
<sm:output value="@{obj.description}" />
</sm:row>
</sm:autocomplete>
<sm:autocomplete id="autocptl-1" var="obj" value="@{autoBean.inputVal}" values="@{autoBean.listValues}"
label="Autocomplete with min length [4]" placeholder="Enter the word here for searching results"
inputText="@{obj.title}" minLength="4" leftAddOn="Search">
<sm:row>
<sm:header title="@{obj.title}" />
<sm:output value="@{obj.description}" />
</sm:row>
</sm:autocomplete>
<sm:autocomplete id="autocptl-2" var="obj" value="@{autoBean.inputVal}" values="@{autoBean.listAdapter}"
label="Autocomplete using list adapter" inputText="@{obj.title}"
placeholder="Enter the word here for searching results" scrollSize="5" maxHeight="200px" leftAddOn="Search">
<sm:row>
<sm:header title="@{obj.title}" />
<sm:output value="@{obj.description}" />
</sm:row>
<sm:load />
</sm:autocomplete>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the autocomplete component |
style | string | false | - | Specifies the css style to be applied to autocomplete component along with Bootstrap input styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to autocomplete component along with Bootstrap input styles. It accepts Expression language and/or text values |
rest | string | false | - | Specifies the JSON key name or XML tag name for REST requests using Rest component. Check Rest component for more details |
length | integer | false | - | Specifies maximum length allowed for text content on autocomplete component |
minLength | integer | false | 1 | Specifies the minimum length of input value to trigger the searching on server via Ajax request |
size | string | false | - | Specifies the Bootstrap size for the autocomplete. Possible values are small,
large
|
var | string | true | - | Specifies the variable name to be used when generating a list of searched results
for the autocomplete component. This value must also be used to access each item on
provided list to capture the values for informed attribute names inside internal
row component.
|
value | string | true | - | Specifies the value for the autocomplete component for partial searching and for
regular requests case this component is inside a form component. It
only accept Expression language
values.
|
values | string | true | - | Specifies the attribute exposed via getter method of type List Collection or ListAdapter to provided the list of content
resulted from search on server using the value informed. It only accept
Expression language values.
|
scrollSize | integer | false | - | Specifies the maximum of elements returned when values attribute uses
the ListAdapter as list provider.
|
scrollOffset | integer | false | - | Specifies the last item value be sent to server when scroll get the bottom of list
to request more items to be searched based on the partial input value. It only
applies when values attribute uses the ListAdapter as list provider.
|
maxHeight | string | false | - | Specifies the maxHeight in pixels to provide a list with scroll element in order to
request more search results when scroll reaches the bottom of list. It only applies
when values attribute uses the ListAdapter
as list provider.
|
readOnly | boolean | false | - | Specifies if the autocomplete component is read only field |
disabled | boolean | false | - | Specifies if the autocomplete component is disabled |
placeholder | string | false | - | Specifies the placeholder to be presented inside the autocomplete input component to easy the field understanding. It accepts Expression language and/or text values |
label | string | false | - | Specifies label for the autocomplete component. It accepts Expression language and/or text values |
leftAddOn | string | false | - | It includes an addon button component or static text on left side of the
autocomplete component. It accepts Expression
language and/or text values. To include a Button
component as leftAddOn the attribute must contain the same id as the
button tag specified as inner tag of autocomplete component
|
rightAddOn | string | false | - | It includes an addon button component or static text on right side of the
autocomplete component. It accepts Expression
language and/or text values. To include a Button
component as rightAddOn the attribute must contain the same id as the
button specified as inner tag of autocomplete component
|
autoFocus | boolean | false | - | Specifies if the autocomplete component must be focused when page is rendered |
tabIndex | integer | false | - | Specifies the tab index for the autocomplete component be focused when page is traversed by tab key |
mask | string | false | - |
Specifies mask to be applied on autocomplete text content. Check the possible
values on
|
update | string | false | - | Specifies id elements to be updated after request completes. The ids must be specified as comma separated values so the HTML elements can be update with request response content. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
List
Why use it?
List component is a elegant solution to provide a dynamic scrolling list of rows for selection. It also integrates with server side via Expression Language to WebBean.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled list component to your page.
What use inside it?
The main and fundamental component to be used inside list is the Row
component to provide a template for creating a list of items. Also this component accept the
Load component to provide load feedback when requesting more
items when scroll reaches the bottom of the list component.
In addition there is the Empty component to provide feedback when no rows are
found on provider. Have a look in the following components for more details.
How to use it?
To integrate the list
component with WebBean
you must specify the Expression language on
the attribute values
which must point to List Collection or to ListAdapter to provide dynamic scrolling content
of objects.
Some other attributes are required such as scrollSize
and
maxHeight
when using ListAdapter to list
objects.
Also the var
attribute must be provided to be used while
iterating to create a list component. In addition the provider for the list of values only
needs to be exposed via getter method.
<sm:list id="list-example-1" values="@{listBean.listValues}" var="obj"
selectValue="@{listBean.selectedValue}">
<sm:row look="@{obj.look}">
<sm:header title="@{obj.header}" />
<sm:output value="@{obj.text}" />
<sm:badge label="@{obj.number}" />
</sm:row>
</sm:list>
<sm:list id="list-example-2" values="@{listBean.listAdapter}" var="obj"
selectValue="@{listBean.selectedValue}" scrollSize="10" maxHeight="270px">
<sm:row>
<sm:header title="@{obj.header}" />
<sm:output value="@{obj.text}" />
<sm:badge label="@{obj.number}" />
</sm:row>
<sm:load type="h4" label=" Loading ..." />
</sm:list>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the list component |
style | string | false | - | Specifies the css style to be applied to list component along with Bootstrap list styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to list component along with Bootstrap list styles. It accepts Expression language and/or text values |
var | string | false | - | Specifies the variable name to be used when generating a list component. This value
must also be used to access each item on provided list to capture the values for
informed attribute names inside internal row component.
|
selectValue | string | false | - | Specifies if the list component can be selected, and in this case the attribute on WebBean of the type of list objects must be exposed via getter and setter. It only accept Expression language values. |
values | string | false | - | Specifies the attribute exposed via getter method of type List Collection or ListAdapter to provided the list of objects to create a list component. It only accept Expression language values. |
scrollSize | integer | false | - | Specifies the maximum of elements returned when values attribute uses
the ListAdapter as list provider.
|
scrollOffset | integer | false | - | Specifies the last item value be sent to server when scroll get the bottom of list
to request more items from list provided. It only applies when values
attribute uses the ListAdapter as list
provider.
|
maxHeight | string | false | - | Specifies the maxHeight in pixels to provide a list with scroll element in order to
request more objects when scroll reaches the bottom of list. It only applies when
values attribute uses the ListAdapter as list provider.
|
rowMode | string | false | section | Specifies the mode do populate the list via JavaScript. Possible values are section,
append . For more details check JavaScript page.
|
update | string | false | - | Specifies id elements to be updated after request completes. The ids must be specified as comma separated values so the HTML elements can be update with request response content. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Row
Why use it?
Row component is meant to be used as inner tag of list
or
autocomplete
components to provide a template for building the list of objects
for those components.
Where to use it?
The row component only takes effect inside the Autocomplete or List component. Check some examples on those component sections.
What use inside it?
This component support any inner components to provide more functionality such as text, output, input, header, badge, button, link, bind events, Ajax request, icon, etc. Have a look in the following components for more details.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the row component used for JavaScript template operations |
style | string | false | - | Specifies the css style to be applied to row component along with Bootstrap row styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to row component along with Bootstrap row styles. It accepts Expression language and/or text values |
look | string | false | - | Specifies the Bootstrap look for the row. The possible values are primary,
success, info, warning, danger and default . It accepts Expression language and/or text
values
|
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Table
Why use it?
Table component is a elegant solution to provide a dynamic scrolling table of rows for selection. It also integrates with server side via Expression Language to WebBean.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled table component to your page.
What use inside it?
The main and fundamental component to be used inside table is the Column component to provide a template for creating a list
of rows. Also this component accept the Load component to
provide load feedback when requesting more rows when scroll reaches the bottom of the table
component.
In addition there is the Empty component to
provide feedback when no rows are found on provider. Have a look in the following components
for more details.
# | Name | Description | Number |
---|---|---|---|
0 | Name 0 | Some description here | 0 |
1 | Name 1 | Some description here | 1 |
2 | Name 2 | Some description here | 2 |
# | Name | Description | Number |
---|---|---|---|
0 | Name 0 | Some description here | 0 |
1 | Name 1 | Some description here | 1 |
2 | Name 2 | Some description here | 2 |
# | Name | Description | Number |
---|---|---|---|
0 | Name 0 | Some description here | 0 |
1 | Name 1 | Some description here | 1 |
2 | Name 2 | Some description here | 2 |
Age | Name | Surname | Points |
---|---|---|---|
33 | Jill | Smith | 150 |
45 | Eve | Jackson | 94 |
25 | John | Doe | 200 |
27 | Adam | Johnson | 115 |
30 | Scarlet | Migre | 149 |
37 | Joel | Garry | 130 |
28 | Clark | Kent | 300 |
18 | Jeniffer | Stinson | 249 |
25 | Steve | Morgan | 100 |
55 | Brad | Perty | 89 |
|
|
|
|
---|---|---|---|
33 | Jill | Smith | 150 |
45 | Eve | Jackson | 94 |
25 | John | Doe | 200 |
27 | Adam | Johnson | 115 |
30 | Scarlet | Migre | 149 |
37 | Joel | Garry | 130 |
28 | Clark | Kent | 300 |
18 | Jeniffer | Stinson | 249 |
25 | Steve | Morgan | 100 |
55 | Brad | Perty | 89 |
<sm:table id="table-example-1" values="@{tableBean.tableValues}" caption="Small static table"
var="obj" selectValue="@{tableBean.selectedValue}" size="small">
<sm:column label="#">
<sm:output value="@{obj.id}" />
</sm:column>
<sm:column label="Name">
<sm:output value="@{obj.name}" />
</sm:column>
<sm:column label="Description">
<sm:output value="@{obj.description}" />
</sm:column>
<sm:column label="Number">
<sm:output value="@{obj.number}" />
</sm:column>
</sm:table>
<sm:table id="table-example-2" values="@{tableBean.tableValues}" caption="Static table"
var="obj" selectValue="@{tableBean.selectedValue}">
<sm:column label="#">
<sm:output value="@{obj.id}" />
</sm:column>
<sm:column label="Name">
<sm:output value="@{obj.name}" />
</sm:column>
<sm:column label="Description">
<sm:output value="@{obj.description}" />
</sm:column>
<sm:column label="Number">
<sm:output value="@{obj.number}" />
</sm:column>
</sm:table>
<sm:table id="table-example-3" values="@{tableBean.tableValues}" caption="Striped bordered table"
var="obj" selectValue="@{tableBean.selectedValue}" striped="true" bordered="true">
<sm:column label="#">
<sm:output value="@{obj.id}" />
</sm:column>
<sm:column label="Name">
<sm:output value="@{obj.name}" />
</sm:column>
<sm:column label="Description">
<sm:output value="@{obj.description}" />
</sm:column>
<sm:column label="Number">
<sm:output value="@{obj.number}" />
</sm:column>
</sm:table>
<sm:table id="table-example-4" values="@{tableBean.tableAdapter}" caption="Dynamic striped table"
striped="true" scrollSize="10" maxHeight="200px" var="obj" selectValue="@{tableBean.selectedValue}">
<sm:column label="Age" styleClass="col-md-2">
<sm:output value="@{obj.age}" />
</sm:column>
<sm:column label="Name" styleClass="col-md-4">
<sm:output value="@{obj.name}" />
</sm:column>
<sm:column label="Surname" styleClass="col-md-4">
<sm:output value="@{obj.surname}" />
</sm:column>
<sm:column label="Points" styleClass="col-md-2">
<sm:output value="@{obj.points}" />
</sm:column>
<sm:load label=" Loading ..." type="h5" />
</sm:table>
<sm:table id="table-example-5" values="@{tableBean.tableAdapter}" caption="Dynamic ordered and filtered table"
scrollSize="10" maxHeight="200px" var="obj" selectValue="@{tableBean.selectedValue}">
<sm:column label="Age" styleClass="col-md-2" sortBy="age" filterBy="age">
<sm:output value="@{obj.age}" />
</sm:column>
<sm:column label="Name" styleClass="col-md-4" sortBy="name" filterBy="name">
<sm:output value="@{obj.name}" />
</sm:column>
<sm:column label="Surname" styleClass="col-md-4" sortBy="surname" filterBy="surname">
<sm:output value="@{obj.surname}" />
</sm:column>
<sm:column label="Points" styleClass="col-md-2" sortBy="points" filterBy="points">
<sm:output value="@{obj.points}" />
</sm:column>
<sm:load label=" Loading ..." type="h5" />
<sm:empty styleClass="doc-empty">
<sm:icon name="glyphicon-glass" />
<sm:output value="No items found" />
</sm:empty>
</sm:table>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the table component |
style | string | false | - | Specifies the css style to be applied to table component along with Bootstrap table styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to table component along with Bootstrap table styles. It accepts Expression language and/or text values |
var | string | false | - | Specifies the variable name to be used when generating a table component. This
value must also be used to access each item on provided list to capture the values
for informed attribute names inside internal column component.
|
selectValue | string | false | - | Specifies if the table component can be selected, and in this case the attribute on WebBean of the type of list objects must be exposed via getter and setter. It only accept Expression language values. |
values | string | false | - | Specifies the attribute exposed via getter method of type List Collection or TableAdapter to provided the list of objects to create a table component. It only accept Expression language values. |
caption | string | false | - | Specifies caption for the table component. It accepts Expression language and/or text values |
bordered | boolean | false | - | Specifies if the table component will present cell border. |
striped | boolean | false | - | Specifies if the table component will present table rows striped. |
size | string | false | - | Specifies the size of the table row. Possible values are small, large
|
scrollSize | integer | false | - | Specifies the maximum of elements returned when values attribute uses
the TableAdapter as list provider.
|
scrollOffset | integer | false | - | Specifies the last item value be sent to server when scroll get the bottom of table
to request more items from list provided. It only applies when values
attribute uses the TableAdapter as list
provider.
|
maxHeight | string | false | - | Specifies the maxHeight in pixels to provide a table with scroll element in order
to request more objects when scroll reaches the bottom of table. It only applies
when values attribute uses the TableAdapter
as list provider.
|
rowMode | string | false | section | Specifies the mode do populate the table via JavaScript. Possible values are section,
append . For more details check JavaScript page.
|
update | string | false | - | Specifies id elements to be updated after request completes. The ids must be specified as comma separated values so the HTML elements can be update with request response content. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Column
Why use it?
Column component is meant to be used as inner tag of table
component to provide
a template for building the table with list of objects.
Where to use it?
The column component only takes effect inside the Table component. Check some examples on those component section.
What use inside it?
This component support any inner components to provide more functionality such as text, output, input, header, badge, button, link, bind events, Ajax request, icon, etc. Have a look in the following components for more details.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the column component |
style | string | false | - | Specifies the css style to be applied to column component along with Bootstrap column styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to column component along with Bootstrap column styles. It accepts Expression language and/or text values |
label | string | true | - | Specifies the header label for the column. It accepts Expression language and/or text values |
sortBy | string | false | - | Specifies the sort order of the table based on var object attribute name. Up and down arrows will be presented to specify the ordering. |
filterBy | string | false | - | Specifies the filter of the table based on var object attribute name. An input will be presented on table header for receiving the value for filtering. |
Empty
Why use it?
Empty component is meant to be used as inner tag of list
, table
or
repeat
components to provide a template for building a feedback message when no
content is found to fill in the component.
Where to use it?
The empty component only take effect inside the List, Table or Empty component. Check some examples on those component sections.
What use inside it?
This component support any inner components to provide more functionality such as text, output, input, header, badge, button, link, bind events, Ajax request, icon, etc. Have a look in the following components for more details.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the empty component used for JavaScript template operations |
style | string | false | - | Specifies the css style to be applied to empty component. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to empty component. It accepts Expression language and/or text values |
Feedback components
JSmart provide a series of feedback components for bringing warning from server side or even working with useful information containers on client side with useful features and pretty styled elements provided via Bootstrap integration.
Alert
Why use it?
Alert component is integrated with WebBean via WebContext to provide intuitive and detailed content response from server side.
Where to use it?
It can be placed anywhere inside body tag in your JSP page and even inside another components to provide pretty styled alert component to your page.
What use inside it?
This component support inner static texts along with other components to provide more functionality such as header, output, button, link, icon etc. Have a look in the following components for more details.
How to use it?
Include the alert component into your JSP page (inside your body tag) and provide an id attribute which is used on WebBean server side via WebContext to send messages or the entire alert content for the id provided when request is completed
Alert info text added via WebContext.addInfo("alert-0", "message");
Alert success text added via WebContext.addSuccess("alert-1", "message");
Alert warning text added via WebContext.addWarning("alert-2", "message");
Header here
Alert error text added via WebContext.addError("alert-3", "message");
Title from bean
Alert info entire content added via WebContext.addAlert("alert-4", new WebAlert(AlertType.INFO));
<sm:alert id="alert-0">
<!-- Content text added here via WebContext -->
</sm:alert`>
<sm:alert id="alert-1" dismissible="false">
<!-- Content text added here via WebContext -->
</sm:alert>
<sm:alert id="alert-2" onHide="alert('On alert hide');">
<!-- Content text added here via WebContext -->
</sm:alert>
<sm:alert id="alert-3">
<sm:header title="Header here">
<sm:icon name="glyphicon-fire"/>
</sm:header>
<!-- Content text added here via WebContext -->
<sm:button label="Alert button" look="danger" />
</sm:alert>
<sm:alert id="alert-4" dismissible="false">
<!-- Entire content added here via WebContext -->
</sm:alert>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the alert component to be accessed via WebBean |
style | string | false | - | Specifies the css style to be applied to alert component along with Bootstrap alert styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to alert component along with Bootstrap alert class. It accepts Expression language and/or text values |
dismissible | boolean | false | true | Shows the close link on top right side of the alert to close it when clicked |
onHide | string | false | - | JavaScript functions to be called before the alert is hidden |
Popover
Why use it?
Popover component can be used to provide content from server side as popover dialog over a specific component when event is triggered by mouse over or mouse click.
Where to use it?
This component is meant to be placed inside other components to provide the popover dialog when the wrapper component is clicked o hovered by mouse.
What use inside it?
This component support any inner components to provide more functionality such as text, output, input, header, badge, button, link, icon, etc. Have a look in the following components for more details.
How to use it?
This component must be placed inside another components. Also this component accepts single
output texts specified via content
attribute but if inner components are found
inside popover
component the content
attribute is ignored.
Click in this label to show the popover
<sm:input label="Input with popover" placeholder="Pass mouse over the input to show the popover">
<sm:popover title="Popover title" event="hover" content="Popover content using attribute only!" />
</sm:input>
<sm:output type="strong" value="Pass mouse over this output to show the popover on top">
<sm:popover title="Popover title" event="hover" content="Popover content on top side!" side="top" />
</sm:output>
<sm:label value="Click in this label to show the popover" style="font-size: 14px;" look="warning">
<sm:popover title="Popover title">
<sm:header>Popover content title</sm:header>
<sm:output>Popover content using output as inner component!</sm:output>
<div align="right">
<sm:button label="Button here" look="warning">
<sm:icon name="glyphicon-user" />
</sm:button>
</div>
</sm:popover>
</sm:label>
<sm:select label="Click on select to show the popover on bottom">
<sm:popover title="Popover title" side="bottom">
<sm:header>Popover content title</sm:header>
<sm:input label="Popover content using input!" placeholder="Popover input ;)" />
<div align="right">
<sm:button label="Button here" look="primary">
<sm:icon name="glyphicon-user" />
</sm:button>
</div>
</sm:popover>
</sm:select>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the popover component |
title | string | false | - | Specifies the title for popover. It accepts Expression language and/or text values |
content | string | false | - | Specifies the content as simple text for popover. It accepts Expression language and/or text values |
event | string | false | click | Specifies the event which should trigger the popover to show or hide. Possible
values are focus, hover, click, manual
|
side | string | false | right | Specifies the side tht the popover must be presented. Possible values are top,
right, bottom, left
|
Tooltip
Why use it?
Tooltip component can be used to provide content from server side as tooltip over a specific component when event is triggered by mouse over or mouse click.
Where to use it?
This component is meant to be placed inside other components to provide the tooltip when the wrapper component is clicked o hovered by mouse.
What use inside it?
This component support any inner components to provide more functionality such as text, output, input, header, badge, button, link, icon, etc. Have a look in the following components for more details.
How to use it?
This component must be placed inside another components and this component only accepts single output texts or components specified inside it.
Click in this label to show the tooltip
<sm:input label="Input with tooltip" placeholder="Pass mouse over the input to show the tooltip">
<sm:tooltip title="Tooltip title">
Tooltip content on right side!
</sm:tooltip>
</sm:input>
<sm:output type="strong" value="Pass mouse over this output to show the tooltip on top">
<sm:tooltip title="Tooltip title" side="top">
Tooltip content on top side!
</sm:tooltip>
</sm:output>
<sm:label value="Click in this label to show the tooltip" style="font-size: 14px;" look="danger">
<sm:tooltip title="Tooltip title" side="top" event="click">
<sm:header>Tooltip content title</sm:header>
<sm:output>Tooltip content using output as inner component!</sm:output>
<div align="right" style="margin-bottom: 5px;">
<sm:button label="Button here" look="danger">
<sm:icon name="glyphicon-user" />
</sm:button>
</div>
</sm:tooltip>
</sm:label>
<sm:select label="Click on select to show the tooltip on bottom">
<sm:tooltip title="Tooltip title" event="click" side="bottom">
<sm:header>Tooltip content title</sm:header>
<sm:input label="Tooltip content using input!" placeholder="Tooltip input ;)" />
<div align="right" style="margin-bottom: 5px;">
<sm:button label="Button here" look="primary">
<sm:icon name="glyphicon-user" />
</sm:button>
</div>
</sm:tooltip>
</sm:select>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the tooltip component |
title | string | false | - | Specifies the title for tooltip. It accepts Expression language and/or text values |
event | string | false | hover | Specifies the event which should trigger the tooltip to show or hide. Possible
values are focus, hover, click, manual
|
side | string | false | right | Specifies the side tht the tooltip must be presented. Possible values are top,
right, bottom, left
|
Load
Why use it?
Load component can be used to easily provide animation and text feedback for any Ajax request to server side using pretty elements provided via Bootstrap integration.
Where to use it?
Include the load component into your JSP page to provide animated and text feedback to Ajax request action to server side. The load can only be included inside the following components.
<sm:link label="Link with loading" action="@{linkBean.doAction}">
<sm:load />
</sm:link>
<sm:button id="btn-load" ajax="true" label="Button with loading" action="@{buttonBean.doAction}">
<sm:load />
</sm:button>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the load component |
style | string | false | - | Specifies the css style to be applied to load component. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to load component. It accepts Expression language and/or text values |
icon | string | false | - | Specifies the Bootstrap glyphicon name to be used as loading animation |
label | string | false | - | Specifies the label for the load component. It accepts Expression language and/or text values |
type | string | false | - | Specifies the size of loading via header HTML element. Possible values are h1,
h2, h3, h4, h5 and h6
|
Progressbar
Why use it?
Progressbar component can be easily used to provide progress feedback of any kind and also it is integrated with server side on WebBean via Expression language.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled progressbar component to your page.
What use inside it?
This component support inner components to provide more functionality such as bind events, Ajax request and tooltip. Have a look in the following components for more details.
<sm:output type="strong" value="Simple progressbar"/>
<sm:progressbar value="50" />
<sm:output type="strong" value="Integrated progressbar"/>
<sm:progressbar value="@{progressBean.progress}" look="success" withLabel="true" />
<sm:output type="strong" value="Progressbar with label and min width"/>
<sm:progressbar value="0" withLabel="true" minWidth="2em" look="warning" />
<sm:output type="strong" value="Progressbar with interval and update via JavaScript"/>
<sm:progressbar value="0" onInterval="updateProgressBar" interval="400" look="info" />
<sm:output type="strong" value="Striped progressbar with interval and update via JavaScript"/>
<sm:progressbar value="15" onInterval="updateProgressBar" interval="400" striped="true" look="success" />
<sm:output type="strong" value="Animated progressbar with label and update via JavaScript"/>
<sm:progressbar value="30" onInterval="updateProgressBar" interval="400" withLabel="true" animated="true" look="danger" />
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the progressbar component |
style | string | false | - | Specifies the css style to be applied to progressbar component along with Bootstrap progressbar styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to progressbar component along with Bootstrap progressbar styles. It accepts Expression language and/or text values |
rest | string | false | - | Specifies the JSON key name or XML tag name for REST requests using Rest component. Check Rest component for more details |
ajax | boolean | false | - | Specifies if the value update for progressbar should use Ajax request on every interval timeout |
value | integer | true | - | Specifies the value for the progressbar component. It accepts Expression language and/or text values. The value must be the type of Integer. |
minValue | integer | false | 0 | Specifies the minimum value for the progressbar component used for calculation. It accepts Expression language and/or text values. The value must be the type of Integer. |
maxValue | integer | false | 100 | Specifies the maximum value for the progressbar component used for calculation. It accepts Expression language and/or text values. The value must be the type of Integer. |
minWidth | integer | false | - | Specifies the minimum width for the progressbar component when value is equal to minValue. |
striped | boolean | false | false | Specifies if the progress bar is striped. |
animated | boolean | false | false | Specifies if the progress bar will present the striped bar animated. |
withLabel | boolean | false | false | Specifies if the progress bar will present the percentage label in the center of bar. |
look | string | false | - | Specifies the Bootstrap look for the progressbar. The possible values are success,
info, warning, danger . It accepts Expression
language and/or text values
|
interval | integer | false | - | Specifies the time interval in milliseconds to check for progressbar
value update. If ajax attribute is true every interval timeout will
trigger an Ajax request to server to get the current progressbar value. Also on every interval the JavaScript function specified on onInterval
attribute is called and the progressbar can be updated directly on client side.
|
onInterval | string | false | - | Specifies a JavaScript function to be called every the interval times out and after
Ajax request is made to server case ajax attribute us true.The function may receive parameters such as element(ProgressBar Object), value(Integer), minValue(Integer) and maxValue(Integer) in that order. Also this function is allowed to return a new value to be set on progressbar. |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Progress group
Why use it?
Progressgroup component can be easily used to provide group of progress feedback of any kind and also it is integrated with server side on WebBean via Expression language.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled progress group component to your page.
What use inside it?
This component was meant to be used along with progressbar
components inside
it, but it support other inner components to provide more functionality such as bind events,
Ajax request and tooltip. Have a look in the following components for more details.
How to use it?
This component works along with inner progressbar
components in which they must
specify a portion of the total value for the group. The total value is automatically
calculated based on each range of each internal progressbar. So be careful when placed the
internal progressbar components which must be placed following the minValue
and
maxValue
order.
<sm:output type="strong" value="Simple progress group"/>
<sm:progressgroup>
<sm:progressbar value="30" minValue="0" maxValue="30" look="success" />
<sm:progressbar value="60" minValue="30" maxValue="60" look="warning" />
<sm:progressbar value="80" minValue="60" maxValue="100" look="danger" />
</sm:progressgroup>
<sm:output type="strong" value="Animated progress group with label"/>
<sm:progressgroup>
<sm:progressbar value="30" minValue="0" maxValue="30" look="info" animated="true" withLabel="true" />
<sm:progressbar value="70" minValue="30" maxValue="70" animated="true" withLabel="true" />
<sm:progressbar value="100" minValue="70" maxValue="100" look="warning" animated="true" withLabel="true" />
</sm:progressgroup>
<sm:output type="strong" value="Progress group with interval"/>
<sm:progressgroup onInterval="updateProgressBar" interval="400">
<sm:progressbar value="0" look="success" minValue="0" maxValue="40" minWidth="2em" />
<sm:progressbar value="0" look="warning" minValue="40" maxValue="80" animated="true" withLabel="true" />
<sm:progressbar value="0" look="danger" minValue="80" maxValue="100" />
</sm:progressgroup>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the progressgroup component |
style | string | false | - | Specifies the css style to be applied to progressgroup component along with Bootstrap progressbar styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to progressgroup component along with Bootstrap progressbar styles. It accepts Expression language and/or text values |
ajax | boolean | false | - | Specifies if the value update for progressbars inside progressgroup should use Ajax request on every interval timeout |
interval | integer | false | - | Specifies the time interval in milliseconds to check for progressbars
values update. If ajax attribute is true every interval timeout will
trigger an Ajax request to server to get the current progressbars values. Also on every interval the JavaScript function specified on onInterval
attribute is called and the progressbar can be updated directly on client side.
|
onInterval | string | false | - | Specifies a JavaScript function to be called every the interval times out and after
Ajax request is made to server case ajax attribute us true.The function may receive parameters such as current element(ProgressBar Object), value(Integer), minValue(Integer) and maxValue(Integer) in that order. Also this function is allowed to return a new value to be set on progressgroup. |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Container components
JSmart provide a series of containers for organizing your page and some integrated with server with useful features and pretty styled elements provided via Bootstrap integration.
Modal
Why use it?
Modal component can be used to easily organize your page and present dynamic content.
Where to use it?
It can be placed anywhere in or JSP page but we recommend you to declare modals at the beginning of of your body tag.
What use inside it?
This component support any inner components to provide more functionality such as text, output, input, header, badge, button, link, bind events, Ajax request, icon, panel, tab, etc. Have a look in the following components for more details.
How to use it?
The use is pretty straight forward, only need its declaration with unique id which can be
used to hide and show the dialog via JavaScript directly. Other components work together
modal to provide more functionality such as the header
and footer
components which are used to header and footer area respectively.
<sm:modal id="modal-example">
<sm:header title="Modal title">
<sm:icon name="glyphicon-modal-window" />
</sm:header>
<p>Here some modal content</p>
<sm:footer>
<sm:button id="hide_button_dialog" label="Hide Modal" look="primary">
<sm:bind event="click" execute="modal-example.hide()" />
</sm:button>
</sm:footer>
</sm:modal>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the modal component |
style | string | false | - | Specifies the css style to be applied to modal component along with Bootstrap modal styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to modal component along with Bootstrap modal styles. It accepts Expression language and/or text values |
opened | boolean | false | - | Specifies if the modal must be presented opened when page is loaded. It accepts Expression language and/or text values |
backdrop | boolean | false | true | Specifies if the modal can be closed when mouse is pressed outside the modal area. |
fade | boolean | false | true | Specifies if the modal uses fade effect when showing or hiding. |
size | string | false | - | Specifies the size of the modal. Possible values are small, large . For
different size please use the style or styleClass to
define the width for the modal
|
onShow | string | false | - | Specifies JavaScript functions to be called before modal is shown. |
onShown | string | false | - | Specifies JavaScript functions to be called after modal is shown. |
onHide | string | false | - | Specifies JavaScript functions to be called before modal is hidden. |
onHidden | string | false | - | Specifies JavaScript functions to be called after modal is hidden. |
Panel
Why use it?
Panel component can be used to easily organize your page and present dynamic content.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled panel component to your page.
What use inside it?
This component support any inner components to provide more functionality such as text, output, input, header, badge, button, link, bind events, Ajax request, icon, panel body, tab, etc. Have a look in the following components for more details.
How to use it?
This component does not require the panelbody
to be specified inside it.
Panel header
Panel header
<sm:panel id="panel-example-1">
<sm:panelbody align="center">
Panel without header nor footer
</sm:panelbody>
</sm:panel>
<sm:panel id="panel-example-2" look="success">
<sm:header id="header_panel" title="Panel header">
<sm:icon name="glyphicon-th-list" />
</sm:header>
<sm:panelbody align="center">
Panel with header
</sm:panelbody>
</sm:panel>
<sm:panel id="panel-example-3" look="danger">
<sm:header id="header_panel" title="Panel header">
<sm:icon name="glyphicon-th-list" />
</sm:header>
<sm:panelbody align="center">
Panel with header and footer
</sm:panelbody>
<sm:footer>
<sm:output value="Panel footer" />
</sm:footer>
</sm:panel>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the panel component |
style | string | false | - | Specifies the css style to be applied to panel component along with Bootstrap panel styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to panel component along with Bootstrap panel styles. It accepts Expression language and/or text values |
look | string | false | - | Specifies the Bootstrap look for the panel. The possible values are primary,
success, info, warning, danger, default . It accepts Expression language and/or text
values
|
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Panel body
Why use it?
Panel body component is meant to be used as inner tag of panel
component to
provide a content for building the panel component.
Where to use it?
The panel body component only takes effect inside the Panel component. Check some examples on those component sections.
What use inside it?
This component support any inner components to provide more functionality such as text, output, input, header, badge, button, link, icon, etc. Have a look in the following components for more details.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the panel body component |
style | string | false | - | Specifies the css style to be applied to panel body component along with Bootstrap panel body styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to panel body component along with Bootstrap panel body styles. It accepts Expression language and/or text values |
type | string | false | - | Specifies the type of panel body. Possible values are fieldset,
section
|
type | string | false | - | Specifies the align of panel body content. Possible values are left, center,
right
|
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Accordion
Why use it?
Accordion component can be used to easily organize your page and present dynamic content using panels dynamically controlled.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled accordion component to your page.
What use inside it?
This component only accepts the Panel inside it to create the accordion with dynamically controlled panels.
<sm:accordion>
<sm:panel look="success">
<sm:header title="Panel one header">
<sm:icon name="glyphicon-th-list" />
</sm:header>
<sm:panelbody align="center">
Panel one content
</sm:panelbody>
</sm:panel>
<sm:panel look="warning">
<sm:header title="Panel two header">
<sm:icon name="glyphicon-th-list" />
</sm:header>
<sm:panelbody align="center">
Content two content
</sm:panelbody>
</sm:panel>
<sm:panel look="danger">
<sm:header title="Panel three header">
<sm:icon name="glyphicon-th-list" />
</sm:header>
<sm:panelbody align="center">
Content three content
</sm:panelbody>
</sm:panel>
</sm:accordion>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the accordion component |
style | string | false | - | Specifies the css style to be applied to accordion component along with Bootstrap panel-group styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to accordion component along with Bootstrap panel-group class. It accepts Expression language and/or text values |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Tab
Why use it?
Tab component can be used to easily organize your page and present dynamic content separated in tabs and sub tabs via dropdown menus.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled tab component to your page.
What use inside it?
This component is meant to be used along with Tab pane components inside it to separate content into tabs.
How to use it?
In order to organize the content into tabs it needs to have inner tabpane
components. Also the tabpane
accepts inner tabpane
components and
in this case it will be organized as dropdown menu.
Pills tab with sub menus
<sm:output type="strong" value="Basic tab with sub menus" />
<sm:tab id="tab-example-1">
<sm:tabpane label="Tab one">
<sm:icon name="glyphicon-glass" />
<div style="margin: 10px; text-align: center;">Tab one content here</div>
</sm:tabpane>
<sm:tabpane label="Tab two">
<sm:icon name="glyphicon-user" />
<sm:tabpane label="Sub tab one" header="User tab section">
<div style="margin: 10px; text-align: center;">Tab two - sub tab one content here</div>
</sm:tabpane>
<sm:tabpane label="Sub tab two" divider="true">
<div style="margin: 10px; text-align: center;">Tab two - sub tab two content here</div>
</sm:tabpane>
<sm:tabpane label="Sub tab three">
<div style="margin: 10px; text-align: center;">Tab two - sub tab three content here</div>
</sm:tabpane>
</sm:tabpane>
<sm:tabpane label="Tab three">
<sm:icon name="glyphicon-star" />
<div style="margin: 10px; text-align: center;">Tab three content here</div>
</sm:tabpane>
</sm:tab>
<sm:output type="strong" value="Pills tab with sub menus" />
<sm:tab id="tab-example-2" pills="regular">
<sm:tabpane label="Tab one">
<sm:icon name="glyphicon-glass" />
<div style="margin: 10px; text-align: center;">Tab one content here</div>
</sm:tabpane>
<sm:tabpane label="Tab two">
<sm:icon name="glyphicon-user" />
<sm:tabpane label="Sub tab one" header="User tab section">
<div style="margin: 10px; text-align: center;">Tab two - sub tab one content here</div>
</sm:tabpane>
<sm:tabpane label="Sub tab two" divider="true">
<div style="margin: 10px; text-align: center;">Tab two - sub tab two content here</div>
</sm:tabpane>
<sm:tabpane label="Sub tab three">
<div style="margin: 10px; text-align: center;">Tab two - sub tab three content here</div>
</sm:tabpane>
</sm:tabpane>
<sm:tabpane label="Tab three">
<sm:icon name="glyphicon-star" />
<div style="margin: 10px; text-align: center;">Tab three content here</div>
</sm:tabpane>
</sm:tab>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the tab component |
style | string | false | - | Specifies the css style to be applied to tab component along with Bootstrap tab styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to tab component along with Bootstrap tab styles. It accepts Expression language and/or text values |
navStyle | string | false | - | Specifies the css style to be applied to nav bar of tab component along with Bootstrap nav styles. It accepts Expression language and/or text values |
navClass | string | false | - | Specifies the css class to be applied to nav bar of tab component along with Bootstrap nav styles. It accepts Expression language and/or text values |
tabValue | string | false | - | Specifies the server side attribute on WebBean to be used for sending and retrieving current tab selected value. It must use Expression language to specify the attribute exposed via getter and setter on WebBean |
ajax | boolean | false | - | Specifies the Ajax request should be triggered when tab is clicked to set value on
WebBean attribute specified via tabValue
|
onShow | string | false | - | Specifies JavaScript functions to be called before selected tab is shown. |
onShown | string | false | - | Specifies JavaScript functions to be called after selected tab is shown. |
onHide | string | false | - | Specifies JavaScript functions to be called before selected tab is hidden. |
onHidden | string | false | - | Specifies JavaScript functions to be called after selected tab is hidden. |
pills | string | false | - | Specifies the type of tab to be presented. Possible values are stacked,
regular . There is also a restriction that justified attribute
with value true cannot be used together the pills with value stacked.
|
justified | boolean | false | - | Specifies if the nav bar of tab will be justified, i.e., if the nav bar will fulfill the entire width space and the tabs will be split evenly |
fade | boolean | false | true | Specifies if the tab uses fade effect when showing or hiding tab panes. |
update | string | false | - | Specifies id elements to be updated after Ajax request completes. The ids must be specified as comma separated values so the HTML elements can be update with request response content. |
beforeSend | string | false | - | JavaScript functions to be called before the Ajax request is made to server. The function may receive parameters such as jqXHR(XmlHttpRequest) and settings(Object). For more details check the link http://api.jquery.com/jquery.ajax |
onError | string | false | - | JavaScript functions to be called if the Ajax request returns error from server. The function may receive parameters such as jqXHR(XmlHttpRequest), textStatus(String) and error(Error). For more details check the link http://api.jquery.com/jquery.ajax |
onSuccess | string | false | - | JavaScript functions to be called if the Ajax request returns successfully from server. The function may receive parameters such as data(Object), textStatus(String) and jqXHR(XmlHttpRequest). For more details check the link http://api.jquery.com/jquery.ajax |
onComplete | string | false | - | JavaScript functions to be called when the Ajax request completes regardless error or success. The function may receive parameters such as jqXHR(XmlHttpRequest) and textStatus(String). For more details check the link http://api.jquery.com/jquery.ajax |
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Tab pane
Why use it?
Tab pane component is meant to be used as inner tag of tab
component to provide
a content for building the tab component.
Where to use it?
The tab pane component only takes effect inside the Tab component. Check some examples on those component sections.
What use inside it?
This component support any inner components to provide more functionality such as text,
output, input, header, badge, button, link, icon, etc.
One important thing to remember
is that the first direct icon
component child is used as tab icon. Have a look
in the following components for more details.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the tab pane component |
style | string | false | - | Specifies the css style to be applied to tab pane content along with Bootstrap tab pane styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to tab pane content along with Bootstrap tab pane styles. It accepts Expression language and/or text values |
tabStyle | string | false | - | Specifies the css style to be applied to tab navigator along with Bootstrap tab styles. It accepts Expression language and/or text values |
tabClass | string | false | - | Specifies the css class to be applied to tab navigator along with Bootstrap tab styles. It accepts Expression language and/or text values |
header | string | false | - | Specifies the header for sub tab dropdown. It accepts Expression language and/or text values |
label | string | true | - | Specifies the label for sub tab dropdown action or for the tab navigator. It accepts Expression language and/or text values |
divider | boolean | false | false | Specifies if the header for sub tab dropdown will present a divider at the bottom side |
disabled | boolean | false | false | Specifies if the sub tab dropdown action or the tab is disabled |
value | string | false | - | Specifies the tab navigator value to be sent to server side when it is clicked case
tab ajax attribute is true. It accepts Expression language and/or text
values
|
This component also accepts mapping HTML events as attributes to execute JavaScript functions. Check General Events for more details.
Carousel
Why use it?
Carousel component can be easily used to present slides of organized content to your JSP page with pretty sytled control and with possibility to quick integration with server side.
Where to use it?
It can be placed anywhere in your JSP page and even inside another components to provide pretty styled carousel component to your page.
What use inside it?
This component is meant to be used along with a combination of internal Slides and/or Slide components.
How to use it?
In order to use carousel
component the internal slides
or slide
components must be present. For using the slides the value
attribute must be
specified to provide a List of SlideAdapter
to fill the slides.
<sm:carousel id="carousel-example-1" width="100%" height="300px" style="margin-bottom: 30px;">
<sm:slide active="true">
<sm:header title="Slide one">
<sm:icon name="glyphicon-picture" />
</sm:header>
<sm:output value="Slide one content text" />
</sm:slide>
<sm:slide>
<sm:header title="Slide two">
<sm:icon name="glyphicon-picture" />
</sm:header>
<sm:output value="Slide two content text" />
</sm:slide>
<sm:slide>
<sm:header title="Slide three">
<sm:icon name="glyphicon-picture" />
</sm:header>
<sm:output value="Slide three content text" />
</sm:slide>
</sm:carousel>
<sm:carousel id="carousel-example-2" width="100%" height="300px" style="margin-bottom: 30px;">
<sm:slide active="true" label="Slide two content label description">
<sm:header title="Slide one - text only">
<sm:icon name="glyphicon-picture" />
</sm:header>
</sm:slide>
<sm:slide imageLib="css.images" imageName="carousel_example.png">
<sm:header title="Slide two - with image">
<sm:icon name="glyphicon-picture" />
</sm:header>
</sm:slide>
<sm:slide imageLib="css.images" imageName="carousel_example.png" label="Slide three content label description ">
<sm:header title="Slide three - with image">
<sm:icon name="glyphicon-picture" />
</sm:header>
</sm:slide>
</sm:carousel>
<sm:carousel id="carousel-example-3" width="100%" height="300px" style="margin-bottom: 30px;" timeout="10000">
<sm:slides values="@{carouselBean.slides}" />
</sm:carousel>
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | false | - | Specifies the id for the carousel component |
style | string | false | - | Specifies the css style to be applied to carousel component along with Bootstrap carousel styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to carousel component along with Bootstrap carousel styles. It accepts Expression language and/or text values |
timeout | integer | false | 5000 | Specifies timeout in milliseconds to change change the slide. If value is zero the timeout will not apply then the slides must be changed manually via side or bottom control |
continuous | boolean | false | true | Specifies if the carousel will restart from first slide when the last slide is reached |
onSlide | string | false | - | Specifies the JavaScript function to be called before slide will be changed |
width | string | false | - | Specifies the width for the slide. It does not need to be specified if all internal
slide
|
height | string | false | - | Specifies the height for the slide. It does not need to be specified if all
internal slide
|
Slide
Why use it?
Slide component is meant to be used as inner tag of carousel
component to
provide a single slide for carousel component.
Where to use it?
The slide component only takes effect inside the Carousel component. Check some examples on those component sections.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
id | string | true | - | Specifies the id for the slide component |
style | string | false | - | Specifies the css style to be applied to slide component along with Bootstrap slide styles. It accepts Expression language and/or text values |
styleClass | string | false | - | Specifies the css class to be applied to slide component along with Bootstrap slide styles. It accepts Expression language and/or text values |
label | string | false | - | Specifies the label to be used as output text on slide body. If this slide uses
imageName attribute the label will be used at the bottom of the slide
right after the title, otherwise it will be placed at the top of slide right after
the title. It accepts Expression
language and/or text values
|
active | boolean | false | - | Specifies if the first slide to be presented when page is loaded. |
imageLib | string | false | - | Specifies the path in which the image is stored inside your webapp folder. It accepts Expression language and/or text values |
imageName | string | false | - | Specifies relative or static image name to be displayed. In case relative name, the lib attribute must be specified, so the image can be located inside your webapp folder. It accepts Expression language and/or text values |
imageAlt | string | false | - | Specifies the alternative name case image cannot be loaded. It accepts Expression language and/or text values |
imageWidth | string | false | - | Specifies the width to be applied on image |
imageHeight | string | false | - | Specifies the height to be applied on image |
Slides
Why use it?
Slides component is meant to be used as inner tag of carousel
component to
provide a List of slides via SlideAdapter for carousel
component.
Where to use it?
The slides component only takes effect inside the Carousel component. Check some examples on those component sections.
Tag attributes
The tag attributes provide more functionality and options for component rendering. Have a look in the possibilities below:
Name | Type | Required | Default | Description |
---|---|---|---|---|
values | string | true | - | Specifies the slides for the carousel component as List Collection
containing SlideAdapter objects. It only
accepts Expression language to map WebBean attribute to provide the
List Collection
|
General Events
Include HTML general event attributes on JSmart components to execute JavaScript when the event is triggered. Most components accept the following the attributes to map events.
Name | Type | Required | Default | Description |
---|---|---|---|---|
onClick | string | false | - | JavaScript functions to be called when component is clicked |
onDblClick | string | false | - | JavaScript functions to be called when component is double clicked |
onMouseDown | string | false | - | JavaScript functions to be called when mouse down is fired on component |
onMouseMove | string | false | - | JavaScript functions to be called when mouse is moved over component |
onMouseOver | string | false | - | JavaScript functions to be called when mouse enter on component |
onMouseOut | string | false | - | JavaScript functions to be called when mouse leave component |
onMouseUp | string | false | - | JavaScript functions to be called when mouse up is fired on component |
onKeyDown | string | false | - | JavaScript functions to be called when key down is fired and the focus is on component |
onKeyPress | string | false | - | JavaScript functions to be called when key is pressed and the focus is on component |
onKeyUp | string | false | - | JavaScript functions to be called when key up is fired and the focus is on component |
onBlur | string | false | - | JavaScript functions to be called when blur is fired and the focus is on component |
onChange | string | false | - | JavaScript functions to be called when change is fired on component |
onFocus | string | false | - | JavaScript functions to be called when focus is fired on component |
onSelect | string | false | - | JavaScript functions to be called when select is done on component |